Supercharge Your WordPress Site’s Speed: Optimizing the Main Thread
July 20, 2023 | By David Selden-Treiman | Filed in: Website Speed.Introduction
Welcome! If you’re here, you’re likely a WordPress developer, enthusiast, or a website owner wondering how to make your site faster and more efficient. Let’s start by addressing the elephant in the room: the main thread. This is the heart of any webpage, where most of the magic happens. But like any good magician, it can get overwhelmed if it’s trying to perform too many tricks at once.
Think of the main thread like a single, incredibly busy waiter at a bustling restaurant. It’s responsible for taking orders (processing JavaScript), serving up delicious dishes (rendering HTML and CSS), and dealing with customer requests (handling user interactions). Now, if that waiter is overwhelmed, the restaurant’s service slows down, and customers aren’t happy.
That’s what happens to your WordPress site when the main thread is overloaded. Pages take longer to load, interactions become sluggish, and your visitors might leave out of frustration. That’s why we need to optimize how the main thread works to improve your website’s performance and keep your visitors happy.
In this article, we’re going to dive deep into the world of main thread work on WordPress. We’ll explore what it is, why it matters, and most importantly, how you can optimize it to boost your website’s performance. We’ll also discuss how web workers can help improve performance by sharing the main thread’s load.
Consider this scenario: Imagine you have a WordPress site that uses a lot of JavaScript. Every time a user visits your site, their browser has to download, parse, compile, and execute that JavaScript. All of this happens on the main thread, and while it’s busy with JavaScript, it can’t do anything else. This can lead to a delay in rendering your site’s content, causing a poor user experience.
// A JavaScript function that could block the main thread
function calculatePrimes(limit) {
let primes = [];
for(let i = 2; i <= limit; i++) {
if(isPrime(i)) {
primes.push(i);
}
}
return primes;
}
// This function checks whether a number is prime - it could take a long time for large numbers
function isPrime(num) {
for(let i = 2, sqrt = Math.sqrt(num); i <= sqrt; i++) {
if(num % i === 0) {
return false;
}
}
return num > 1;
}
calculatePrimes(1000000); // This line could block the main thread
In this example, the calculatePrimes
function could take a significant amount of time for large inputs, during which it would block the main thread. This could lead to a noticeable delay in rendering your website.
By the end of this article, you’ll have a good understanding of how to avoid these issues and optimize the main thread work on your WordPress site. So, let’s get started!
Understanding Main Thread Work
To optimize main thread work, it’s vital to first understand what exactly is happening on the main thread. In the simplest terms, the main thread is where your browser does most of its work, such as parsing HTML to construct the Document Object Model (DOM), executing JavaScript, and handling user interactions.
What Is the Main Thread?
In the context of a web browser, the main thread handles the majority of the processing. It’s like the main character in a movie, always at the center of the action. For WordPress, this involves parsing the HTML of your website, creating the DOM, and executing any JavaScript code.
Think of the main thread as an assembly line where the raw materials (HTML, CSS, JavaScript) are processed into a finished product (a rendered webpage). Just like an assembly line, if one part of the process takes too long, everything else is held up, which slows down the whole production process.
How Does the Main Thread Work?
One of the key roles of the main thread is executing JavaScript. When a webpage is loaded, the browser reads the HTML from top to bottom. Whenever it encounters a script tag, it stops to download, parse, and execute that JavaScript before continuing. This is known as “parser blocking,” because the HTML parser has to stop for the JavaScript.
For example, consider this HTML snippet:
<!DOCTYPE html>
<html>
<head>
<title>My Website</title>
<script src="big-script.js"></script>
</head>
<body>
<!-- The rest of your website's content -->
</body>
</html>
In this example, the browser starts parsing the HTML. When it reaches the script
tag, it stops to download big-script.js
, parse it, and execute it. Only after it’s done with all that will it continue parsing the rest of the HTML. If big-script.js
is large or complex, this can lead to a noticeable delay in displaying your website’s content.
What Happens When the Main Thread is Slow?
When the main thread is overloaded, it can lead to a sluggish user experience. This is because while the main thread is busy with one task, it can’t handle other tasks. For example, if it’s busy executing a large JavaScript file, it can’t respond to user interactions, which can make your website feel slow or unresponsive.
Imagine you have a WordPress site with a lot of complex JavaScript animations. While those animations might look cool, they can put a heavy load on the main thread. If a user tries to interact with your site while the main thread is busy with those animations, their interactions might be delayed or ignored, leading to a frustrating user experience.
By understanding the role and behavior of the main thread, you can make better decisions about how to structure and optimize your WordPress site. In the next section, we’ll look at some common causes of heavy main thread work and how to address them.
Common Causes of Heavy Main Thread Work in WordPress
Now that you’ve got a handle on what the main thread does and why it’s so important, let’s delve into what might be weighing it down on your WordPress site. By pinpointing these common culprits, you’ll be better equipped to lighten the load and speed up your site.
Inefficient JavaScript Handling
JavaScript is a powerful tool, but when used inefficiently, it can bog down the main thread. This usually happens when you have large blocks of JavaScript code, complex calculations, or scripts that load synchronously, thereby blocking the parser.
For instance, you might have a script that dynamically loads images based on user interactions. If not optimized, this script could slow down your website, particularly if it’s loading large images or if many images are being loaded at once.
// This script loads an image whenever a button is clicked
let button = document.getElementById('load-image-button');
button.addEventListener('click', function() {
let img = new Image();
img.src = 'large-image.jpg';
document.body.appendChild(img);
});
In this case, every time the button is clicked, a large image is loaded and appended to the body of the document. If the image is very large or the button is clicked multiple times, this could put a heavy load on the main thread.
Excessive DOM Manipulations
Every time a change is made to the DOM, the browser has to recalculate the layout of the page, which can be computationally expensive. If your WordPress site frequently updates the DOM, such as adding, removing, or changing elements, it can slow down the main thread.
Consider a WordPress site that uses a lot of dynamic content, like a news site that constantly updates headlines or a weather site that frequently updates temperature and weather conditions. Each of these updates can cause a change in the DOM, forcing the browser to recalculate the layout.
Unoptimized Media Files
Media files, especially images and videos, can be large and take a long time to download and render. Every time the browser encounters a media file, it has to stop and download it before it can continue rendering the page. If your WordPress site uses a lot of media files or doesn’t properly optimize them, it can slow down the main thread.
For instance, you might have a gallery of high-resolution images. While these images might look great, they can also be very large. Each time a user visits your site, their browser has to download and render these large images, which can slow down the main thread.
Bloated WordPress Themes
Some WordPress themes come with a lot of built-in features and functionalities. While these can be useful, they can also add a lot of extra JavaScript and CSS that has to be processed by the main thread. If your theme is bloated with features you don’t use, it can slow down your site.
Excessive Use of Plugins
Plugins are a great way to add functionality to your WordPress site, but each plugin also adds more work for the main thread. Every plugin comes with its own JavaScript and CSS, and potentially even more if it adds new elements to the DOM. If you use a lot of plugins, or use plugins that are not well optimized, they can put a heavy load on the main thread.
Understanding these common causes of heavy main thread work can help you identify potential issues on your WordPress site. In the next section, we’ll dive into specific techniques to minimize main thread work and boost your site’s performance.
Techniques to Minimize Main Thread Work
With a solid understanding of what can slow down the main thread, you’re ready to tackle optimization. Here are some practical techniques to minimize main thread work on your WordPress site.
Optimizing JavaScript
Improving the way your site handles JavaScript can significantly reduce the load on the main thread.
Minifying and compressing scripts
Minification removes unnecessary characters (like whitespace and comments) from your code, making it smaller and faster to download. Compression can further reduce the file size.
// Before minification
function addNumbers(num1, num2) {
let result = num1 + num2;
return result;
}
// After minification
function addNumbers(n,r){return n+r;}
You can use WordPress plugins or build tools like UglifyJS for minification.
Deferring non-critical JavaScript
Deferring a script prevents it from blocking the parser. It tells the browser to continue building the DOM and only execute the script once that’s done.
<script src="my-script.js" defer></script>
Using asynchronous loading
Similar to deferring, asynchronous loading tells the browser to continue parsing the HTML while the script is being downloaded. It then executes the script as soon as it’s ready, even if the browser hasn’t finished building the DOM.
<script src="my-script.js" async></script>
Reducing DOM Complexity
Reducing the complexity of your DOM can make your site faster and more efficient.
- Removing unnecessary elements: Every element in your DOM adds to its complexity. If you have elements that aren’t needed, removing them can speed up your site.
- Optimizing CSS selectors: Complex CSS selectors can slow down your site because the browser has to work harder to determine which elements the selector applies to. Try to keep your selectors as simple as possible.
/* Before optimization */
div.content > ul.list > li.item > a.link { color: blue; }
/* After optimization */
.link { color: blue; }
Optimizing Media Files
Large media files can slow down the main thread. Here’s how you can optimize them:
- Compressing images and videos: Compression reduces the file size of your images and videos without significantly reducing their quality. You can use tools like Photoshop for images and Handbrake for videos.
- Using lazy loading: Lazy loading is a technique where you delay loading of images or other content until they’re needed. For instance, images further down the page can be loaded only when the user scrolls near them.
<img data-src="my-image.jpg" class="lazyload">
You can use JavaScript or a WordPress plugin to implement lazy loading.
Selecting and Maintaining WordPress Themes and Plugins
The themes and plugins you use can greatly impact the main thread work.
- Choosing lightweight themes: Some themes are built with performance in mind. They come with less CSS and JavaScript, meaning less work for the main thread.
- Limiting plugin use: Each plugin adds more work for the main thread. Evaluate if each plugin you use is necessary, or if there are more efficient alternatives.
- Regularly updating and reviewing themes and plugins: Developers frequently update their themes and plugins to improve performance. Make sure you’re using the latest versions, and periodically review your theme and plugin usage.
With these techniques, you can significantly reduce the workload of your main thread and improve the performance of your WordPress site. But we’re not done yet! In the next section, we’ll look at how web workers can offload some of this work from the main thread.
Leveraging Web Workers for Performance Improvement
Web Workers are a powerful tool that can take your website performance to the next level. They allow you to run JavaScript in the background, on a separate thread, freeing up the main thread to do what it does best – dealing with user interactions and rendering your webpage.
What are Web Workers?
Web Workers are a simple yet powerful concept. They allow you to offload tasks that would normally block the main thread onto a separate thread. This can significantly improve the responsiveness and performance of your website.
How Web Workers Can Offload Main Thread Work
Web Workers run in the background and can handle tasks such as:
- Performing computations in the background: Instead of blocking the main thread with heavy calculations, you can offload them to a Web Worker.
- Handling network requests: Web Workers can fetch data from the network without blocking the main thread.
Integrating Web Workers in a WordPress Site
Now, let’s see how to create and use a Web Worker:
Creating a Web Worker
A Web Worker is created by calling the Worker()
constructor with a JavaScript file as an argument.
let worker = new Worker('worker.js');
Sending messages between the main thread and the Web Worker
You can use the postMessage()
method to send messages between the main thread and the Web Worker, and the onmessage
event handler to receive them.
// In the main thread
let worker = new Worker('worker.js');
worker.postMessage('Hello, worker!');
worker.onmessage = function(event) {
console.log('Received from worker: ' + event.data);
};
// In worker.js
self.onmessage = function(event) {
console.log('Received from main thread: ' + event.data);
self.postMessage('Hello, main thread!');
};
Handling errors in Web Workers
You can use the onerror
event handler to catch and handle errors that occur in a Web Worker.
worker.onerror = function(error) {
console.log('Error in worker: ' + error.message);
};
Potential Challenges and Limitations of Using Web Workers
While Web Workers can significantly improve your website’s performance, they do come with some challenges and limitations:
- Web Workers can’t directly manipulate the DOM: Since they run on a separate thread, Web Workers don’t have access to the DOM. If a task involves updating the DOM, you’ll need to send a message back to the main thread to do it.
- Debugging can be more complex: Because Web Workers run in the background and on a separate thread, debugging them can be more complex than debugging code on the main thread.
- Not all browsers support Web Workers: While most modern browsers support Web Workers, some older versions do not. You’ll need to provide a fallback or a graceful degradation for these browsers.
Even with these challenges, Web Workers can be a powerful tool in your performance optimization toolkit. By offloading heavy tasks to a Web Worker, you can keep your main thread free to provide a smooth and responsive user experience.
WordPress Tools and Plugins for Main Thread Optimization
To help you streamline your WordPress site’s performance, there’s a host of tools and plugins at your disposal. These resources can automate many of the techniques we’ve discussed and make your optimization journey much smoother.
Available Tools and Plugins
WordPress has a vibrant community that has created a plethora of performance optimization tools and plugins. From image optimization to JavaScript minification, there’s a tool for almost every need.
How to Effectively Use These Tools for Main Thread Optimization
Here are a few examples of tools and plugins that can help you minimize main thread work:
- Autoptimize: This plugin can help you optimize your site’s JavaScript, CSS, and HTML. It combines, minifies, and compresses your scripts and styles, making them faster to download and reducing the work your main thread has to do.
<!-- Before Autoptimize -->
<script src="script1.js"></script>
<script src="script2.js"></script>
<!-- After Autoptimize -->
<script src="autoptimized.js"></script>
- Imagify: This plugin can compress your images without losing quality, making them faster to download and reducing the load on the main thread.
- WP Super Cache: This plugin generates static HTML versions of your pages, reducing the need for PHP and MySQL and making your pages faster to load.
- WP Asset Clean Up: This plugin scans your pages and detects any unnecessary CSS or JavaScript that’s being loaded. You can then choose to unload these assets, reducing the work your main thread has to do.
These tools and plugins are just the tip of the iceberg. There are many more out there, and finding the right ones for your specific needs can make a big difference in your site’s performance. Remember, the goal is to keep your main thread as free as possible so it can quickly respond to user interactions and render your site.
In the final section, we’ll summarize everything we’ve learned and look at the bigger picture of performance optimization in WordPress.
Optimizing the Example Code from the Introduction
Let’s revisit the JavaScript code we discussed in the introduction. As you recall, the function calculatePrimes
finds all the prime numbers up to a given limit. While this function works correctly, it could potentially block the main thread for a significant amount of time, especially for large inputs.
// A JavaScript function that could block the main thread
function calculatePrimes(limit) {
let primes = [];
for(let i = 2; i <= limit; i++) {
if(isPrime(i)) {
primes.push(i);
}
}
return primes;
}
// This function checks whether a number is prime - it could take a long time for large numbers
function isPrime(num) {
for(let i = 2, sqrt = Math.sqrt(num); i <= sqrt; i++) {
if(num % i === 0) {
return false;
}
}
return num > 1;
}
calculatePrimes(1000000); // This line could block the main thread
How can we optimize this code to minimize its impact on the main thread? One way is to move this computation to a Web Worker. As we discussed earlier, a Web Worker allows you to run JavaScript in the background, on a separate thread, freeing up the main thread.
Here’s how you can modify the code to use a Web Worker:
Creating the Web Worker
First, create a new JavaScript file for the Web Worker. We’ll call it prime-worker.js
. Move the calculatePrimes
and isPrime
functions to this file.
//prime-worker.js
self.onmessage = function(event) {
let limit = event.data;
let primes = calculatePrimes(limit);
self.postMessage(primes);
};
function calculatePrimes(limit) {
let primes = [];
for(let i = 2; i <= limit; i++) {
if(isPrime(i)) {
primes.push(i);
}
}
return primes;
}
function isPrime(num) {
for(let i = 2, sqrt = Math.sqrt(num); i <= sqrt; i++) {
if(num % i === 0) {
return false;
}
}
return num > 1;
}
Using the Web Worker
In your main JavaScript file, create a new Web Worker and use it to calculate the primes. The main thread sends a message to the Web Worker with the postMessage
method. The message contains the limit up to which we want to calculate primes. The Web Worker then sends a message back to the main thread with the result.
// main.js
let worker = new Worker('prime-worker.js');
worker.postMessage(1000000); // Send the limit to the worker
worker.onmessage = function(event) {
console.log(event.data); // Log the primes received from the worker
};
worker.onerror = function(error) {
console.log('Error in worker: ' + error.message);
};
With this setup, the heavy computation of finding prime numbers is offloaded to the Web Worker, allowing the main thread to remain responsive to user interactions. This is just one example of how you can optimize your JavaScript code to minimize main thread work and improve the performance of your WordPress site. For a production site, you’d also want to minify this code and serve if from a cache to optimize performance.
Conclusion
Congratulations! You’ve embarked on an important journey to optimize the main thread of your WordPress site. You’ve learned why it’s important, what can slow it down, and how to speed it up.
We’ve discussed how the main thread is like the star of the show, the main character in your website’s story. It has the important job of parsing HTML to build the DOM, executing JavaScript, and responding to user interactions. But when it gets overwhelmed with too much to do, it can’t perform at its best, leading to a slow, frustrating user experience.
To help our star performer, we’ve learned techniques such as optimizing JavaScript, reducing DOM complexity, compressing media files, and selecting efficient WordPress themes and plugins. We’ve also seen how to offload some of the work to web workers, freeing up the main thread to do what it does best.
But remember, performance optimization is not a one-time task. It’s an ongoing process that requires continuous learning and adaptation as web technologies and best practices evolve. So, stay curious, keep experimenting, and always strive to make your WordPress site faster and more efficient.
In the world of website performance, even a small improvement can make a big difference. So, take what you’ve learned today, apply it to your WordPress site, and make your main thread the best it can be. Your users will thank you!
Thank you for joining us on this journey. We hope you found this guide helpful and informative. Keep exploring, keep learning, and keep optimizing!
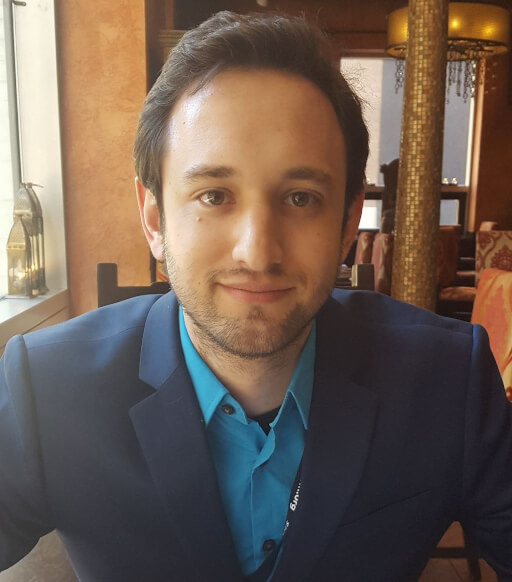
David Selden-Treiman is Director of Operations and a project manager at Potent Pages. He specializes in custom web crawler development, website optimization, server management, web application development, and custom programming. Working at Potent Pages since 2012 and programming since 2003, David has extensive expertise solving problems using programming for dozens of clients. He also has extensive experience managing and optimizing servers, managing dozens of servers for both Potent Pages and other clients.
Comments are closed here.