Javascript Worklets API: Optimizing Your WordPress Site in 2024
July 21, 2023 | By David Selden-Treiman | Filed in: Website Speed.As a WordPress user, you’ve probably faced one challenge that we all strive to overcome – optimizing website performance. Your site’s speed and responsiveness have significant implications on user experience, SEO, and ultimately your website’s success. That’s where JavaScript, the language that powers interactivity on the web, comes into play. It’s an incredibly powerful tool when used effectively, and it can seriously supercharge your WordPress site’s performance.
Today, we’re going to talk about a particularly fascinating part of JavaScript known as the Worklets API. Maybe you’ve heard of it, maybe you haven’t. Regardless, by the end of this article, you’ll not only understand what it is but also how to implement it to get your WordPress site running at peak performance.
The JavaScript Worklets API opens up exciting avenues for optimizing your site. It’s like adding a turbocharger to your website engine, significantly boosting speed and responsiveness. It offers an effective way to write code that runs off the main thread, leading to much smoother user experiences.
Picture this: you’ve got a beautiful WordPress blog about travel, full of vibrant photos, engaging animations, and immersive audio clips. But when users visit, they’re left waiting for the site to load, the animations stutter, and the audio lags. With the Worklets API, these issues can be a thing of the past. This powerful JavaScript feature lets you handle rich media and animations more efficiently, keeping your audience engaged and coming back for more.
Now, sit tight and buckle up as we take a deep dive into the world of WordPress performance optimization with the JavaScript Worklets API. Whether you’re a seasoned developer or a curious beginner, there’s something for everyone. Let’s begin our journey towards a more streamlined, speedy WordPress website!
Background and Importance
Over the years, website optimization has become more than a luxury—it’s a necessity. Slow load times and laggy interfaces can be the difference between a user sticking around or leaving for a competitor’s website. After all, nobody likes waiting around, right?
Let’s imagine your WordPress site as a bustling restaurant. Your content is the delicious food that you serve, while the overall performance and speed of your site are akin to the service in your restaurant. Even if you have the most delectable dishes, if the service is slow and inefficient, your customers might not stick around. The same principle applies to your WordPress site.
Traditionally, we’ve used various methods to speed up WordPress sites—using optimized themes, compressing images, enabling caching, minimizing HTTP requests, and so on. While these methods are still effective and valuable, the web’s dynamic nature demands even more. As web technologies advance, we get the tools to make our sites not only faster but also more interactive and engaging. This is where JavaScript and the Worklets API come into play.
In terms of SEO, website performance is crucial. Search engines like Google place a great deal of importance on a site’s load time, among other performance metrics. The faster your site, the better your chances of ranking higher in search engine results. And let’s not forget the users. A fast, responsive website contributes significantly to a positive user experience, leading to higher visitor retention and conversion rates.
That’s where the JavaScript Worklets API comes in. It’s a next-generation tool that lets you enhance your site’s performance on multiple levels, from how it loads and renders content to how it processes and presents audio.
Consider the Worklets API as the secret ingredient to your WordPress site’s success recipe. You might have great content (the main course), a beautiful design (the presentation), but without optimized performance (the perfect seasoning), your site might just fall short of winning your visitors’ hearts (or in this case, clicks).
So, ready to take your WordPress site to the next level with the JavaScript Worklets API? Let’s delve deeper and explore what it is and how it can turbocharge your WordPress site!
What is the JavaScript Worklets API?
The JavaScript Worklets API is an exciting feature that allows you to write high-performance, off-main-thread operations. Think of it as a way to delegate certain tasks to a separate thread, freeing up the main one and making your site run smoother and faster. Worklets operate at a lower level than other web APIs, allowing you more control and flexibility.
Now, the Worklets API isn’t just one single thing—it’s actually a family of APIs, each with a specific purpose. Let’s break them down one by one.
Paint Worklet
Ever wanted to add some custom graphics or visual effects to your site but felt limited by the CSS properties available? The Paint Worklet is your magic wand! It allows you to define custom CSS paint functions, which can be used to draw in any CSS image property like background-image
.
Imagine you want to add a rainbow gradient background that’s not available in standard CSS. With the Paint Worklet, you can create it! Here’s an example:
registerPaint('rainbow', class {
paint(ctx, geom) {
const gradient = ctx.createLinearGradient(0, 0, geom.width, geom.height);
gradient.addColorStop(0, 'red');
gradient.addColorStop(0.15, 'orange');
gradient.addColorStop(0.3, 'yellow');
gradient.addColorStop(0.5, 'green');
gradient.addColorStop(0.65, 'blue');
gradient.addColorStop(0.8, 'indigo');
gradient.addColorStop(1, 'violet');
ctx.fillStyle = gradient;
ctx.fillRect(0, 0, geom.width, geom.height);
}
});
CSS.paintWorklet.addModule('rainbow.js');
Then, in your CSS file, you can call your custom paint worklet:
body {
background-image: paint(rainbow);
}
And voila! You’ve got a custom rainbow gradient background.
Layout Worklet
Layout Worklet allows you to define your own layout algorithms. Ever struggled with a layout that just doesn’t exist in CSS, or wished you could create a custom one that perfectly suits your content? Layout Worklet to the rescue!
Let’s say you want a diagonal layout for a set of elements. You could create a layout worklet like this:
registerLayout('diagonal', class {
async layout(children, edges, constraints, styleMap) {
const childFragments = await Promise.all(children.map((child) => {
return child.layoutNextFragment({});
}));
let offset = 0;
for(let fragment of childFragments) {
fragment.inlineOffset = offset;
fragment.blockOffset = offset;
offset += fragment.blockSize;
}
return {autoBlockSize: offset, childFragments};
}
});
CSS.layoutWorklet.addModule('diagonal.js');
In your CSS, use it like this:
.container {
display: layout(diagonal);
}
This will arrange the children of the .container
element in a diagonal layout.
Animation Worklet
With the Animation Worklet, you can create smoother, jank-free animations, even under heavy loads. It lets you write imperative animations that run at the device’s native frame rate. This means your animations look silky smooth, no matter how complex they are!
Suppose you have an animation that moves an element across the screen over a period of time. The code might look like this:
registerAnimator('moveAcross', class {
animate(currentTime, effect) {
effect.localTime = currentTime;
}
});
CSS.animationWorklet.addModule('moveAcross.js');
In your CSS, you can trigger the animation like this:
.element {
animation: move 2s infinite;
animation-timing-function: linear;
}
@keyframes move {
0% { transform: translateX(0); }
100% { transform: translateX(100vw); }
}
This will animate the element, moving it across the screen.
Audio Worklet
Lastly, the Audio Worklet provides a low-level JavaScript API for processing and synthesizing audio on the web. Want to create a custom audio player on your site with unique effects or filters? The Audio Worklet makes it possible!
Here’s an example of how you could create a simple gain control:
class GainProcessor extends AudioWorkletProcessor {
static get parameterDescriptors() {
return [{ name: 'gain', defaultValue: 1 }];
}
process(inputs, outputs, parameters) {
const input = inputs[0];
const output = outputs[0];
const gain = parameters.gain;
for (let channel = 0; channel < input.length; ++channel) {
const inputChannel = input[channel];
const outputChannel = output[channel];
for (let i = 0; i < inputChannel.length; ++i)
outputChannel[i] = inputChannel[i] * gain[i];
}
return true;
}
}
registerProcessor('gain-processor', GainProcessor);
You can then use this custom processor in your audio context:
const audioContext = new AudioContext();
audioContext.audioWorklet.addModule('gainProcessor.js').then(() => {
const gainNode = new AudioWorkletNode(audioContext, 'gain-processor');
gainNode.connect(audioContext.destination);
});
This creates a gain node that can be used to control the volume of your audio content.
Each of these Worklets is a powerful tool to optimize and customize your WordPress site, creating a unique, engaging, and performant experience for your users. Ready to get started? Let’s get down to the nuts and bolts of using JavaScript Worklets API in WordPress in the next section!
How to Use JavaScript Worklets API in WordPress
Using the JavaScript Worklets API in WordPress isn’t as intimidating as it might initially seem. With a bit of patience and a dash of creativity, you’ll soon have your WordPress site running smoother and faster. Let’s dive in!
Prerequisites
Before getting started, ensure your WordPress site is set up to handle JavaScript properly. This involves enqueuing your JavaScript files in your theme’s or child theme’s functions.php
file using wp_enqueue_script()
.
Implementing the JavaScript Worklets API
Let’s start with how to integrate each type of worklet in your WordPress site.
Paint Worklet
Say you’ve written a custom paint worklet that creates a fun, confetti background. Here’s how you can integrate it into your WordPress site:
- Create a new JavaScript file, let’s call it
confettiWorklet.js
, in your theme’s js directory with your paint worklet code.
registerPaint('confetti', class {
paint(ctx, geom) {
// Your code for creating a confetti background goes here.
}
});
- Enqueue this file in your theme’s
functions.php
:
function enqueue_worklet_scripts() {
wp_enqueue_script('confetti-worklet', get_template_directory_uri() . '/js/confettiWorklet.js', array(), '1.0.0', true);
}
add_action('wp_enqueue_scripts', 'enqueue_worklet_scripts');
- Finally, load the worklet and use it in your CSS:
body {
background-image: paint(confetti);
}
@supports (background: paint(confetti)) {
body {
background-image: paint(confetti);
}
}
Your WordPress site now has a fun confetti background!
Layout Worklet
Implementing a layout worklet in your WordPress site is a similar process.
- Create a new JavaScript file, for example,
customLayoutWorklet.js
, with your layout worklet code.
registerLayout('customLayout', class {
// Your custom layout code goes here.
});
- Enqueue the script:
function enqueue_worklet_scripts() {
wp_enqueue_script('custom-layout-worklet', get_template_directory_uri() . '/js/customLayoutWorklet.js', array(), '1.0.0', true);
}
add_action('wp_enqueue_scripts', 'enqueue_worklet_scripts');
- Then, call your worklet in your CSS:
.container {
display: layout(customLayout);
}
And there you go! Your elements within the .container
will now follow your custom layout.
Animation Worklet
Similarly, to use an animation worklet:
- Create a JavaScript file, say,
smoothScrollWorklet.js
, with your animation worklet code.
registerAnimator('smoothScroll', class {
// Your animation worklet code goes here.
});
- Enqueue the script:
function enqueue_worklet_scripts() {
wp_enqueue_script('smooth-scroll-worklet', get_template_directory_uri() . '/js/smoothScrollWorklet.js', array(), '1.0.0', true);
}
add_action('wp_enqueue_scripts', 'enqueue_worklet_scripts');
- Finally, call the worklet in your CSS:
.element {
animation: smoothScroll 2s infinite;
animation-timing-function: linear;
}
Your animations should now be running silky smooth!
Audio Worklet
Finally, to implement an audio worklet:
- Create a JavaScript file,
customAudioWorklet.js
, with your audio worklet code.
class CustomAudioProcessor extends AudioWorkletProcessor {
// Your custom audio processing code goes here.
}
registerProcessor('custom-audio-processor', CustomAudioProcessor);
- Enqueue the script:
function enqueue_worklet_scripts() {
wp_enqueue_script('custom-audio-worklet', get_template_directory_uri() . '/js/customAudioWorklet.js', array(), '1.0.0', true);
}
add_action('wp_enqueue_scripts', 'enqueue_worklet_scripts');
- Now, you can use this custom processor in your audio context in your regular JavaScript files:
const audioContext = new AudioContext();
audioContext.audioWorklet.addModule('customAudioWorklet.js').then(() => {
const customNode = new AudioWorkletNode(audioContext, 'custom-audio-processor');
customNode.connect(audioContext.destination);
});
You’ve now successfully incorporated custom audio processing in your WordPress site!
Using the Worklets API may look a bit complex at first, but once you start experimenting and get the hang of it, it’ll become a powerful tool in your WordPress development toolkit. In the next section, we’ll dive deeper into specific optimization techniques using Worklets API. Ready to continue the journey? Let’s go!
Optimization Techniques Using Worklets API
Once you’ve got the basics of the Worklets API down, you can start exploring various optimization techniques to make your WordPress site faster, more responsive, and more efficient. Let’s take a look at a few of these techniques.
Reducing Main Thread Load with Worklets
One of the key benefits of the Worklets API is its ability to perform operations off the main thread. This is particularly valuable because the main thread is where most of the browser’s work happens — parsing HTML, executing JavaScript, calculating layouts, painting pixels, and so on. By offloading some tasks to worklets, you can keep the main thread free and responsive.
Let’s take a paint worklet, for example. Say your website has a dynamic background that changes based on user interaction, and this is implemented in JavaScript running on the main thread. With a paint worklet, you can offload this task to the worklet.
Here’s a simplified example:
registerPaint('dynamicBackground', class {
paint(ctx, geom, properties) {
const interaction = properties.get('--user-interaction')[0];
// paint the background based on interaction
}
});
CSS.paintWorklet.addModule('dynamicBackground.js');
In your CSS:
body {
background-image: paint(dynamicBackground);
--user-interaction: 0; /* this will be updated in your JavaScript */
}
This way, even if your main thread is busy with other tasks, your dynamic background will continue to run smoothly because it’s being handled by the paint worklet on a separate thread.
Creating Responsive Layouts with Layout Worklet
Layout worklets can help you create responsive layouts that adjust automatically based on the viewport size, the size of the parent container, or other factors. For example, you could create a layout worklet that arranges items in a grid with a dynamic number of columns based on the container width.
registerLayout('responsiveGrid', class {
async layout(children, edges, constraints, styleMap) {
const inlineSize = constraints.fixedInlineSize;
const childInlineSize = 100; // assuming each child is 100px wide
const numColumns = Math.floor(inlineSize / childInlineSize);
// layout code that arranges children in a grid with numColumns columns
}
});
CSS.layoutWorklet.addModule('responsiveGrid.js');
In your CSS:
.container {
display: layout(responsiveGrid);
}
This way, your layout will automatically adjust to the available space without the need for media queries or JavaScript running on the main thread.
Enhancing User Interaction with Animation Worklet
Animation worklets can significantly enhance user interaction on your site by providing smooth, jank-free animations. For example, you could create an animation worklet for a smooth scroll effect that responds to user scrolling with a parallax effect.
registerAnimator('parallaxScroll', class {
constructor(options) {
this.speed = options.speed;
}
animate(currentTime, effect) {
effect.localTime = currentTime * this.speed;
}
});
CSS.animationWorklet.addModule('parallaxScroll.js');
In your CSS:
.background {
animation: parallax 1s infinite linear;
animation-play-state: paused;
}
@keyframes parallax {
0% { transform: translateY(0); }
100% { transform: translateY(-100px); } /* adjust based on your needs */
}
In your JavaScript:
document.addEventListener('scroll', () => {
const scrollPosition = window.pageYOffset;
document.querySelector('.background').style.animationPlayState = 'running';
document.querySelector('.background').style.animationDelay = `-${scrollPosition}px`;
});
This will create a parallax scroll effect that runs smoothly even under heavy load thanks to the animation worklet.
Custom Audio Processing with Audio Worklet
Finally, the audio worklet allows you to create custom audio effects, synthesis, and processing. For example, you could create an audio worklet that applies a custom reverb effect:
class ReverbProcessor extends AudioWorkletProcessor {
// reverb processing code goes here
}
registerProcessor('reverb-processor', ReverbProcessor);
You can then use this custom processor in your audio context:
const audioContext = new AudioContext();
audioContext.audioWorklet.addModule('reverbProcessor.js').then(() => {
const reverbNode = new AudioWorkletNode(audioContext, 'reverb-processor');
reverbNode.connect(audioContext.destination);
});
This way, you can create unique, high-quality audio experiences on your WordPress site.
User Interface Smoothness with Animation Worklet
Animation worklets can make scrolling and user interface animations much smoother, creating a more enjoyable user experience. For example, you could create an “infinite scroll” effect, where content seamlessly loads as the user scrolls down, using an animation worklet.
registerAnimator('infiniteScroll', class {
animate(currentTime, effect) {
const scrollPosition = window.pageYOffset;
effect.localTime = scrollPosition;
}
});
CSS.animationWorklet.addModule('infiniteScroll.js');
In your CSS:
.content {
animation: infiniteScroll 1s infinite linear;
animation-play-state: paused;
}
In your JavaScript:
document.addEventListener('scroll', () => {
document.querySelector('.content').style.animationPlayState = 'running';
});
This will provide a smoother scrolling experience as new content is loaded.
Enhancing Responsive Images with Paint Worklet
Paint worklets can also be used to enhance responsive images. For instance, you could use a paint worklet to dynamically apply a color filter based on the user’s preferred color scheme.
registerPaint('colorFilter', class {
paint(ctx, geom, properties) {
const userPrefersDark = properties.get('--prefers-dark')[0];
// apply a light or dark filter based on userPrefersDark
}
});
CSS.paintWorklet.addModule('colorFilter.js');
In your CSS:
img {
background-image: paint(colorFilter);
--prefers-dark: matchMedia('(prefers-color-scheme: dark)').matches;
}
This way, your images will automatically adjust to the user’s preferred color scheme without the need for multiple image files or JavaScript running on the main thread.
Loading Scripts Efficiently with Worklets
The way you load your worklet scripts can also improve the performance of your WordPress site. Worklet scripts can be added in an asynchronous way, meaning that the browser does not need to pause the parsing of the HTML document to fetch and execute the script.
For example, let’s say you have an audio worklet script that you want to load:
const audioContext = new AudioContext();
audioContext.audioWorklet.addModule('audioWorklet.js')
.then(() => {
const audioWorkletNode = new AudioWorkletNode(audioContext, 'audio-processor');
audioWorkletNode.connect(audioContext.destination);
});
By using the addModule
method, the worklet script is fetched and executed asynchronously, improving the initial loading speed of your site.
Remember, the key to optimizing your WordPress site with worklets is to take tasks off the main thread and onto separate threads, thereby allowing your site to remain responsive even under heavy load. Always look for opportunities to offload tasks from the main thread to worklets, and your users will thank you for the smooth and responsive experience.
Final Thoughts and Wrap Up
Well, there you have it, an in-depth look at how you can harness the power of the JavaScript Worklets API to optimize your WordPress website.
By now, you’ve got a good sense of what worklets are, why they matter, and how they work. You’ve seen first-hand how worklets can help offload tasks from the main thread, freeing it up for more critical operations and enhancing the performance of your website.
Remember, worklets aren’t a magic fix for all performance issues. They are, however, a powerful tool in your optimization toolkit, capable of dramatically enhancing user experiences by smoothing out animations, accelerating load times, and providing customized audio effects.
To get started, remember these key points:
- Understand Your Needs: Before jumping straight into worklets, make sure you understand what performance issues you’re trying to address. Is it sluggish animations? Slow page loads? A lack of responsiveness in your layout? Once you know what the problem is, you can target it more effectively with worklets.
- Start Small and Iterate: When implementing worklets for the first time, start with a small, manageable project. Get the hang of how worklets work, and once you’re comfortable, expand your usage.
- Test, Test, and Test Again: Always test your implementation thoroughly. Make sure it’s working as expected and improving performance without causing any side effects.
Incorporating worklets into your WordPress site can be a game-changer in terms of improving performance. They allow you to take advantage of multi-threaded operations, opening up new possibilities for improving your site’s responsiveness and overall user experience.
A Final Example
Here’s a final example of how you can use an animation worklet to create a more interactive and engaging user experience:
// Creating an animation worklet for a subtle hover effect
registerAnimator('hoverEffect', class {
animate(currentTime, effect) {
const mouseX = effect.getInputProperties().get('--mouse-x')[0];
const mouseY = effect.getInputProperties().get('--mouse-y')[0];
effect.localTime = calculateDistance(mouseX, mouseY); // you would define calculateDistance function based on your needs
}
});
CSS.animationWorklet.addModule('hoverEffect.js');
In your CSS:
.button {
animation: hoverEffect 1s infinite linear;
animation-play-state: paused;
}
In your JavaScript:
document.querySelector('.button').addEventListener('mousemove', (e) => {
document.documentElement.style.setProperty('--mouse-x', e.clientX);
document.documentElement.style.setProperty('--mouse-y', e.clientY);
document.querySelector('.button').style.animationPlayState = 'running';
});
Now it’s over to you. We can’t wait to see how you use worklets to take your WordPress site to new heights. Don’t be afraid to experiment and push the boundaries – that’s where the real magic happens!
Happy coding, and here’s to a faster, smoother, and more responsive WordPress site!
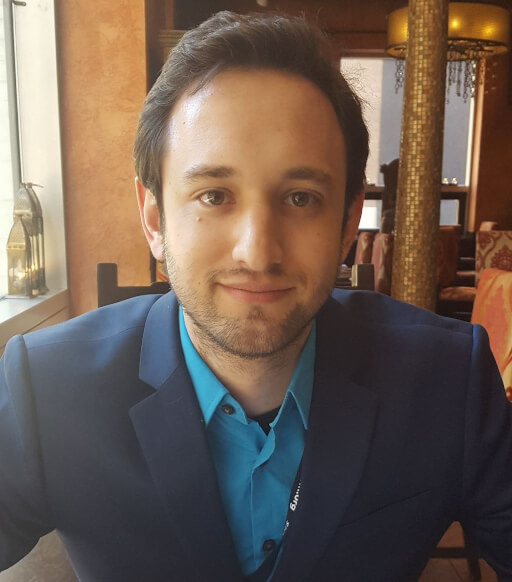
David Selden-Treiman is Director of Operations and a project manager at Potent Pages. He specializes in custom web crawler development, website optimization, server management, web application development, and custom programming. Working at Potent Pages since 2012 and programming since 2003, David has extensive expertise solving problems using programming for dozens of clients. He also has extensive experience managing and optimizing servers, managing dozens of servers for both Potent Pages and other clients.
Comments are closed here.