How to Improve Web Worker Performance In 2024
July 20, 2023 | By David Selden-Treiman | Filed in: Website Speed.Introduction
Hello there, curious reader! If you’ve stumbled upon this guide, chances are you’re interested in how to optimize the performance of web workers. Perhaps you’re building a high-performance web application, or maybe you’re just seeking to learn more about the hidden power of the web’s parallel processing capabilities. Regardless of your motivation, you’ve come to the right place.
What are Web Workers?
Let’s start at the very beginning. A web worker, in the simplest terms, is a script that runs in the background. This script is independent of any user interfaces and allows you to perform tasks that don’t interfere with the user’s interaction with your webpage.
Think of it this way: imagine you’re a chef in a busy restaurant (your web application) and you’ve got a lot of orders (tasks) to process. Now, you could try to handle all of these orders yourself, but you’re only one person, and eventually, you’re going to get overwhelmed. Enter web workers – they’re like your kitchen helpers, working in the background, chopping vegetables, and stirring sauces so you can focus on grilling that steak to perfection.
Here’s a simple example of how to create a dedicated web worker:
let myWorker = new Worker("worker.js");
In the above line of code, “worker.js” is a JavaScript file that contains the tasks you want the web worker to perform. Just like that, you’ve got an extra set of hands to help with your tasks!
Why Do Web Workers Need Optimization?
Now, you might ask, “Why do I need to optimize the performance of my web workers?” Well, unoptimized web workers can lead to several problems like memory leaks, sluggish user interfaces, or just generally inefficient applications. In contrast, a well-optimized web worker contributes to the smooth running of your application, providing a better user experience, making efficient use of resources, and saving processing power.
Optimizing your web workers is like teaching your kitchen helpers to be more efficient. Maybe they could chop the vegetables faster, or perhaps they could stir the sauce while also keeping an eye on the oven. With more efficient helpers, you can process more orders and keep your customers (users) happy and satisfied.
In the upcoming sections, we’re going to dive deeper into the strategies and techniques you can employ to turn your web workers into well-oiled machines. Get ready to roll up your sleeves and dive into the exciting world of web worker optimization!
Understanding the Basics of Web Workers
Before we get into the nitty-gritty of optimization, let’s get familiar with some basics. Just as a good chef should know all the tools in the kitchen, you, as a web developer, should know the different types of web workers and understand when to use them.
Types of Web Workers
Web workers come in three main types: Dedicated Web Workers, Shared Web Workers, and Service Workers. Here’s a quick rundown:
Dedicated Web Workers
These are the most basic type of web workers. They’re like private chefs working on a specific task for a single webpage. Once created, they can only communicate with the main thread that created them.
Here’s how you create and communicate with a dedicated web worker:
let myWorker = new Worker('worker.js');
myWorker.postMessage([first.value, second.value]); // send data to our worker
myWorker.onmessage = function(e) {
console.log('Message received from worker', e.data);
}
In this example, worker.js
is the script that our worker runs. We communicate with the worker using the postMessage
function and receive messages from the worker using the onmessage
event handler.
Shared Web Workers
These workers are shared across multiple instances of the same webpage. They’re like communal chefs, cooking for a whole party of people. They can communicate with any script from the same domain that connects to them.
Creating and communicating with a shared web worker looks like this:
let mySharedWorker = new SharedWorker('worker.js');
mySharedWorker.port.start();
mySharedWorker.port.postMessage([first.value, second.value]);
mySharedWorker.port.onmessage = function(e) {
console.log('Message received from shared worker', e.data);
}
Service Workers
These are a special type of worker that can control web pages, intercept network requests, and cache responses. They’re like a maitre d’, managing communications between the kitchen (your server) and your customers (users).
Setting up a service worker involves a bit more code, so we’ll cover that in detail in a later section.
When to Use Web Workers
Now, understanding when to use web workers is crucial. Just as you wouldn’t use a sledgehammer to crack a nut, there are tasks that are best suited for the main thread and others that are perfect for web workers.
Web workers shine when it comes to computationally heavy tasks that don’t need to interact with the DOM. Examples include number crunching, complex calculations, and data processing tasks.
For instance, if you need to apply a complex filter to an image or calculate the 10000th Fibonacci number, these tasks can be offloaded to a web worker to keep your main thread free and responsive.
On the other hand, tasks like updating the user interface, listening for user interactions, or any tasks that require direct interaction with the web page’s Document Object Model (DOM) should stay on the main thread.
Now that we have a handle on the basics, we can delve into the principles of optimizing these amazing web workers. Onward to performance improvement!
Key Principles of Web Workers Optimization
Well done on reaching this far! Now that we’ve equipped you with the basics, it’s time to get down to the heart of the matter: optimizing your web workers. As we venture into this, think of it as fine-tuning your kitchen, arranging everything for maximum efficiency, so you spend less time reaching for ingredients and more time cooking.
Minimizing the Overhead
Creating a web worker isn’t free; there’s some overhead involved. Every time a new worker is spun up, it takes up memory and processing resources. It’s akin to hiring a new kitchen helper; they’ll need space, equipment, and time to become productive.
One way to reduce this overhead is by reusing workers. Instead of creating a new worker every time you have a task, you can keep a worker running and send it new tasks as needed.
Here’s an example of a web worker being reused for multiple tasks:
let myWorker = new Worker('worker.js');
// Send task 1
myWorker.postMessage({task: 'task1', data: [first.value, second.value]});
// Send task 2
myWorker.postMessage({task: 'task2', data: [third.value, fourth.value]});
myWorker.onmessage = function(e) {
console.log('Message received from worker', e.data);
}
In the above code, we’re sending different tasks to the same worker.
Efficient Communication between Main Thread and Workers
Communication between your main thread and your workers is another area where we can optimize. Remember, every message sent or received is like a note passed between you and your kitchen helper. If the notes are too long or complicated, things can slow down.
When you pass a message to a web worker, the data in the message is copied rather than shared. However, copying large amounts of data can be slow and consume a lot of memory.
To avoid this, you can use Transferable Objects, which are moved rather than copied, making the transfer much faster. But remember, once transferred, the original array is no longer usable. It’s a bit like giving your only chopping board to your helper; they can use it, but you can’t until it’s given back.
Here’s how you would use Transferable Objects:
let myArrayBuffer = new ArrayBuffer(8);
myWorker.postMessage(myArrayBuffer, [myArrayBuffer]);
SharedArrayBuffer is another technique where you can share memory between the main thread and web workers, but as of my knowledge cutoff in September 2021, it’s important to note that SharedArrayBuffer was disabled in many browsers due to security concerns. Always check the current browser support when considering these optimizations.
Task Partitioning
Task partitioning is all about breaking down large tasks into smaller, manageable ones. Imagine trying to cook a three-course meal all at once versus cooking each course one at a time. The latter is much more manageable and will keep your kitchen (or in this case, your application) running smoothly.
The idea is to distribute tasks among several workers. For example, if you have a large array to process, rather than sending the whole array to one worker, you could send chunks of the array to several workers.
Utilization of Service Workers for Caching
Service workers have a unique capability: they can intercept network requests and cache responses. This is like having a well-organized pantry where your ingredients (data) are stored for easy access, saving you trips to the market (network requests).
Here’s a simple example of using a service worker to cache a response:
self.addEventListener('fetch', function(event) {
event.respondWith(
caches.match(event.request)
.then(function(response) {
// Cache hit - return response
if (response) {
return response;
}
return fetch(event.request);
}
)
);
});
In the above code, every time a fetch request is made, the service worker first checks the cache. If a cached response is available, it’s returned, otherwise, a network request is made.
That’s it for this section! By keeping these principles in mind, you can optimize your web workers for maximum performance. Remember, even small improvements can lead to big gains over time. On to the next section, where we’ll delve deeper into techniques and best practices for web worker optimization!
Performance Optimization Techniques and Best Practices
Fantastic progress! Now that we’ve uncovered the key principles of web worker optimization, it’s time to further refine your understanding. Let’s move on to some tried-and-tested techniques and best practices to truly master web worker performance. It’s like discovering a secret recipe to make your cooking even more delicious!
Leveraging Worker Pools
A worker pool is a group of web workers that stand ready to perform tasks as they come in. They’re like a team of chefs, each waiting to take on the next order. Worker pools are an efficient way to reuse workers and minimize the overhead of creating and destroying workers.
You can create a worker pool by keeping track of several workers in an array and assigning them tasks based on their availability.
Here’s a simplified example:
let workerPool = [];
let MAX_WORKERS = 4; // Adjust based on your needs
// Create the pool
for(let i = 0; i < MAX_WORKERS; i++) {
workerPool[i] = new Worker('worker.js');
}
// Use the pool
let workerIndex = 0;
function assignTaskToWorker(taskData) {
workerPool[workerIndex++ % MAX_WORKERS].postMessage(taskData);
}
In this code, we first create a pool of workers. Then, when we need to assign a task, we cycle through the workers in the pool, spreading the tasks evenly.
Appropriate Use of Asynchronous Processing
Web workers thrive on asynchronous processing. They can carry out tasks in the background while your main thread continues to respond to user interactions. It’s like having your kitchen helper prepare the sauce while you’re grilling the steak.
This means you should design your web workers to operate asynchronously. JavaScript Promises and async/await are great tools for managing asynchronous operations.
myWorker.onmessage = function(e) {
console.log('Message received from worker', e.data);
}
async function runTask() {
let result = await new Promise((resolve, reject) => {
myWorker.postMessage([first.value, second.value]);
myWorker.onmessage = e => resolve(e.data);
myWorker.onerror = reject;
});
console.log(result);
}
runTask();
In this code, we wrap our worker’s message handling in a Promise. This allows us to use async/await to write cleaner, easier-to-read code.
Profiling and Debugging Web Workers
Remember, even the best chefs taste their food while they’re cooking. Profiling and debugging your web workers allows you to understand how your workers are performing and where bottlenecks might be occurring.
Most modern browsers’ developer tools allow you to inspect web workers. You can look at the messages being passed, time their operations, and even use breakpoints to pause execution and inspect the state of your worker.
console.time('workerTask');
myWorker.postMessage([first.value, second.value]);
myWorker.onmessage = function(e) {
console.log('Message received from worker', e.data);
console.timeEnd('workerTask');
}
Here, we’re using console.time
and console.timeEnd
to measure how long a task takes to run in the worker.
Error Handling in Web Workers
In any kitchen, spills and accidents happen. The same goes for programming. Effective error handling in your web workers is crucial for robust and reliable applications.
Web workers have an onerror
event that you can use to catch and handle any errors that occur during their execution.
javascriptCopy codemyWorker.onerror = function(e) {
console.log('Error from worker:', e.message);
}
In this code, we’re setting up an error handler that will log any errors from our worker.
Well done! You’re on your way to becoming a master of web worker optimization. Remember, it’s a continuous process of learning, experimenting, and refining. The next section will look at how web workers interact with modern JavaScript frameworks. Let’s keep the momentum going!
Web Workers with Modern JavaScript Frameworks
Kudos for your persistence! By now, you’re well-versed with the basics and advanced principles of optimizing web workers. But the web development world isn’t just about vanilla JavaScript, is it? It’s filled with a smorgasbord of frameworks and libraries, each offering a unique flavor to the dish we call web development.
In this section, we’ll explore how web workers interact with modern JavaScript frameworks like React, Vue, and Angular, and how to optimize their performance in these environments.
Web Workers with React
React is known for its virtual DOM that optimizes rendering, but that doesn’t mean web workers can’t further improve performance. You can use web workers in React to offload computationally heavy tasks, keeping your components responsive.
Here’s a simplified example of using web workers in a React component:
import React, { useEffect, useState } from 'react';
const MyComponent = () => {
const [workerResult, setWorkerResult] = useState(null);
useEffect(() => {
const myWorker = new Worker('worker.js');
myWorker.postMessage('Heavy computation');
myWorker.onmessage = e => setWorkerResult(e.data);
// Clean up
return () => {
myWorker.terminate();
};
}, []);
return (
<div>
{workerResult ? `Result from worker: ${workerResult}` : 'Working...'}
</div>
);
};
export default MyComponent;
In the example above, we’re creating a web worker, sending it a message, and updating our component’s state with the result.
Web Workers with Vue.js
In Vue.js, you can create and use web workers similarly to improve performance. You can create a web worker, send it tasks, and update your Vue instance’s data when the task is complete.
Here’s how you might do that:
<template>
<div>
{{ workerResult ? `Result from worker: ${workerResult}` : 'Working...' }}
</div>
</template>
<script>
export default {
data() {
return {
workerResult: null,
};
},
created() {
const myWorker = new Worker('worker.js');
myWorker.postMessage('Heavy computation');
myWorker.onmessage = e => {
this.workerResult = e.data;
};
},
};
</script>
In the Vue.js example, we’re creating a web worker in the created
lifecycle hook and updating our component’s data with the result from the worker.
Web Workers with Angular
Similarly, in Angular, you can use web workers to run computationally heavy tasks in the background, keeping your Angular components responsive. Here’s an example:
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-my-component',
template: `
<div>
{{ workerResult ? 'Result from worker: ' + workerResult : 'Working...' }}
</div>powerful performance enhancements
`
})
export class MyComponent implements OnInit {
workerResult: any;
ngOnInit() {
const myWorker = new Worker('worker.js');
myWorker.postMessage('Heavy computation');
myWorker.onmessage = ({ data }) => {
this.workerResult = data;
};
}
}
In the Angular example, we’re creating a web worker in the ngOnInit
lifecycle hook and updating our component’s property with the result from the worker.
There you go! By incorporating web workers into your JavaScript frameworks, you’ll not only leverage the optimization principles you’ve learned so far, but also take advantage of the unique benefits these frameworks offer.
Next up, we’ll round things off with some final thoughts on the journey of optimizing web worker performance. You’re almost at the finish line!
Final Thoughts and Future Outlook
Bravo! You’ve sailed through the journey of understanding and optimizing web workers, even extending them to modern JavaScript frameworks. But remember, learning to cook doesn’t end the moment you prepare your first meal. It’s a continuous process of honing your skills and tasting new recipes. Similarly, the world of web workers and web performance is ever-evolving, and staying up-to-date is key. Let’s wrap up with some final thoughts and a look towards the future.
Continual Learning and Adaptation
The field of web development is continuously evolving, with new techniques, technologies, and best practices emerging all the time. As a web developer, you should continually learn and adapt, trying new recipes, so to speak. Stay updated with the latest developments in the field, participate in communities, and don’t shy away from experimenting with new ideas.
Performance Metrics and Benchmarking
In your optimization journey, it’s crucial to keep track of your performance metrics and regularly benchmark your application. Utilize the built-in tools in your browser, or third-party tools, to measure performance and identify bottlenecks. This is like taste-testing your dishes or timing your cooking to ensure you’re improving.
Future of Web Workers
The world of web development is bustling with innovation. New APIs and capabilities are being introduced that could change how we use web workers. For instance, the emerging Worklets API allows you to run small JavaScript snippets on a separate thread, similar to web workers, but with a more specific purpose, like manipulating CSS properties or audio samples.
Another exciting area is WebAssembly (WASM), a low-level binary format that runs at near-native speed. It opens up the possibility for web workers to run more complex and performance-intensive tasks than ever before.
While exploring these advanced topics, always remember the principles and techniques you’ve learned in this article. They will continue to be the foundation upon which you build your knowledge and skills.
That’s it, friend! You’ve now traversed the winding roads of web worker optimization. But remember, this isn’t the end, but rather the beginning of your journey. Don’t be afraid to get your hands dirty and try out what you’ve learned. After all, it’s by trying that we truly learn. Go ahead and cook up some incredible web performance with your newfound knowledge. Bon appétit!
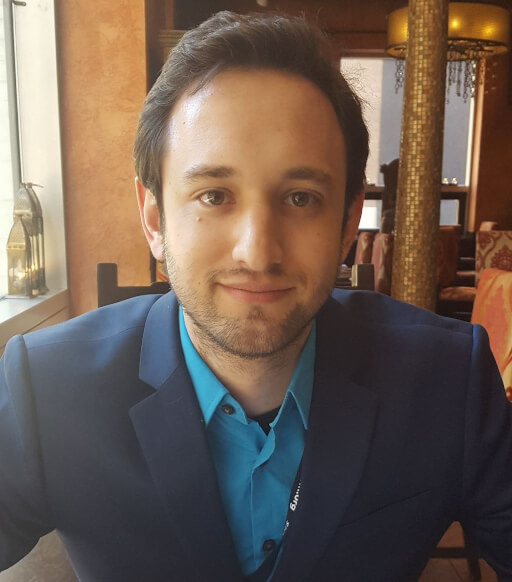
David Selden-Treiman is Director of Operations and a project manager at Potent Pages. He specializes in custom web crawler development, website optimization, server management, web application development, and custom programming. Working at Potent Pages since 2012 and programming since 2003, David has extensive expertise solving problems using programming for dozens of clients. He also has extensive experience managing and optimizing servers, managing dozens of servers for both Potent Pages and other clients.
Comments are closed here.