The Web Storage API: Local & Session Storage in 2025
July 26, 2023 | By David Selden-Treiman | Filed in: Javascript.Welcome! Today we’re going to explore the exciting world of the Web Storage API. If you’ve ever wondered about how websites remember your preferences, or why you don’t have to log into your favorite sites every time you visit, you’re in the right place.
The Web Storage API is a modern solution to storing data in a user’s browser. It’s a little bit like a treasure chest where websites can store things they want to remember for later. You can think of it like the memory of a website, a place where it can store things about you or your visit to recall later.
So where did this all start? The Web Storage API was introduced as part of the HTML5 standard in the late 2000s. Before this, most sites relied on something called cookies to remember things. While cookies did a decent job, they had their limitations, such as a maximum storage size of only 4KB. The Web Storage API was designed to overcome these limitations, and it has a much higher limit – up to 5MB or even more in some browsers!
As web development has advanced, the ability to create sophisticated, app-like experiences in the browser has become increasingly important. The Web Storage API plays a vital role in enabling this, as it allows for much larger and more complex amounts of data to be stored in the browser, compared to what was possible with cookies.
For example, consider an online shopping site. If you add an item to your cart, then navigate away from the page, how does the site remember what was in your cart when you come back? Or perhaps, you’ve customized the site to show prices in your local currency, or to display in dark mode. How does the site remember your preferences for the next time you visit? The answer is the Web Storage API.
Stay with us as we dive deeper into the Web Storage API, its uses, and how you can leverage it to create richer, more engaging experiences for your site’s visitors. We’ll cover everything from the basics to security considerations and best practices. Whether you’re a seasoned developer looking to refresh your knowledge, or a beginner eager to learn, this comprehensive guide will give you all the tools you need to harness the power of the Web Storage API. Let’s get started!
The Basics of the Web Storage API
Understanding the Concept of Web Storage
Before we dive into the nitty-gritty, let’s first understand what web storage really is. In simple terms, web storage allows web applications to store data persistently in a user’s browser. Just like how you save files on your computer to access them later, web storage allows websites to do the same thing. And this isn’t just a little bit of data – depending on the user’s browser, up to 5MB or more can be stored.
Differences Between Web Storage API and Cookies
You might be wondering, “Don’t cookies do the same thing?” That’s a great question. Cookies and the Web Storage API are similar in that they both store data on the user’s browser. However, there are key differences:
- Storage Limit: Cookies can only store up to 4KB of data. The Web Storage API, on the other hand, allows you to store much larger amounts of data (up to 5MB or more).
- Lifecycle: Cookies can persist for a long time, even when the browser is closed, unless they are manually deleted. However, data stored with the Web Storage API can be either persistent or tied to the session, depending on which type you use.
- Transmission to server: Each time a browser makes a request to a server, it includes all cookies from that site with the request. This is not the case with web storage, which can result in improved performance.
Introduction to Two Types of Web Storage: Local Storage and Session Storage
The Web Storage API provides two methods of storage, each with its own use-cases.
- Local Storage: This keeps your data stored even after the browser is closed and reopened. For example, if a user logs in on a site and chooses to stay logged in, their login status could be stored in local storage. When they come back later and reopen the browser, the site can check local storage and see that they’re still logged in.
// Saving data to local storage
localStorage.setItem('key', 'value');
// Getting data from local storage
let myData = localStorage.getItem('key');
- Session Storage: This only keeps the data for one session. This means when the user closes the tab or browser, the data gets deleted. Imagine you’re in the middle of filling out a form on a website, and you accidentally refresh the page. If the site saved your progress in session storage, you wouldn’t lose what you’d filled out so far.
javascriptCopy code// Saving data to session storage
sessionStorage.setItem('key', 'value');
// Getting data from session storage
let myData = sessionStorage.getItem('key');
Limitations of Web Storage API
Like all technologies, the Web Storage API is not without its limitations. The most obvious one is the storage limit. While much larger than cookies, the storage space is still finite. Also, it only works on the client’s browser, meaning you can’t directly access the data from your server.
Furthermore, the Web Storage API is synchronous, which means it can potentially lock the browser’s main thread if it’s doing a lot of work. This could lead to performance issues if not properly managed. Lastly, because the data is stored on the user’s computer, there can be security concerns, especially if sensitive data is stored. We’ll cover more about this in a later section.
Don’t worry about these limitations too much, though. With the right knowledge and techniques, many of these concerns can be effectively managed, and the Web Storage API can become an invaluable tool in your web development toolbox.
Why is the Web Storage API Used?
You’ve now got a solid understanding of what the Web Storage API is and its basic principles. But why do we use it? What benefits does it bring to web development? Let’s explore some reasons.
Why Developers Prefer Web Storage API Over Cookies
As we discussed earlier, the Web Storage API overcomes several limitations of cookies. With more space to store data, the Web Storage API opens up a lot of possibilities for creating dynamic, personalized web experiences. Also, unlike cookies, data stored in the Web Storage API doesn’t get sent to the server with every request, helping to improve website performance. In essence, it gives developers a better toolbox to create more sophisticated web applications.
Use of the Web Storage API for Maintaining Application States
One key use of the Web Storage API is to maintain application state. What does this mean? Imagine you’re halfway through an online survey and need to step away. When you come back, you don’t want to start over from the beginning, right? This is where the Web Storage API comes in handy. It can store your progress, so when you return, you can pick up right where you left off.
Here’s a simple example with JavaScript:
// Let's say this is your progress in the survey
let surveyProgress = {
question1: 'Answer1',
question2: 'Answer2',
question3: 'Answer3'
}
// Before you leave the page, your progress is stored in session storage
sessionStorage.setItem('surveyProgress', JSON.stringify(surveyProgress));
// When you return, the site can check if there's any progress saved
let savedProgress = JSON.parse(sessionStorage.getItem('surveyProgress'));
The Role of Web Storage API in Personalizing User Experiences
Another significant role of the Web Storage API is enabling personalized experiences. Have you ever wondered how a website remembers your preferences, like choosing a dark or light theme, your preferred language, or recently viewed products? The answer is often the Web Storage API.
For instance, if a user selects a dark theme for your website, you can store that preference and apply it whenever they visit your site:
// When a user selects a dark theme
localStorage.setItem('theme', 'dark');
// Now, whenever the user visits, you can check their preferred theme
let theme = localStorage.getItem('theme');
if (theme === 'dark') {
// Apply the dark theme to your website
}
Improving the Performance of Web Applications Using Web Storage API
The Web Storage API can also contribute to improved web performance. Remember, unlike cookies, data stored using the Web Storage API isn’t sent to the server with every request. This can significantly reduce the amount of data sent between the client and server, leading to faster load times and a smoother user experience.
As you can see, the Web Storage API can do a lot to make your web applications more user-friendly, dynamic, and efficient. And best of all, it’s relatively simple to use. But don’t just take our word for it – let’s dive into how you can use it in the next section!
How to Use the Web Storage API
Now that you understand the why, let’s delve into the how. Implementing the Web Storage API is surprisingly straightforward, and once you know the basics, you’ll find it’s a versatile tool for many different scenarios.
How to Use Local Storage
Using local storage involves primarily three operations: storing data, retrieving data, and removing data. Here’s how you can do each:
Storing Data
To store data in local storage, you use the setItem
method. This method accepts two parameters: a key and a value. The key is a string you use to identify the item, and the value is the data you want to store.
Let’s say you’re building a simple to-do app and you want to save the user’s to-dos in local storage. Here’s how you might do it:
let todos = ['Buy milk', 'Walk the dog', 'Read a book'];
localStorage.setItem('todos', JSON.stringify(todos));
Note that we’ve used JSON.stringify
to turn the array into a string before storing it. This is because web storage can only store strings. If you want to store other types of data like arrays or objects, you need to convert them into a string first.
Retrieving Data
To retrieve data from local storage, you use the getItem
method, which accepts one parameter: the key of the item you want to retrieve.
Continuing with our to-do app example, here’s how you might retrieve the saved to-dos:
let savedTodos = JSON.parse(localStorage.getItem('todos'));
Notice the use of JSON.parse
. Since we stored our array as a string, we need to convert it back to an array when we retrieve it.
Deleting Data
To delete a specific item from local storage, you can use the removeItem
method, which accepts the key of the item you want to delete as a parameter.
localStorage.removeItem('todos');
Clearing All Data
To clear all data from local storage, you can use the clear
method.
localStorage.clear();
How to Use Session Storage
Session storage works exactly like local storage, with the only difference being that session storage data disappears when the browser or tab is closed. The methods to store, retrieve, and delete data are the same.
// Storing data in session storage
sessionStorage.setItem('key', 'value');
// Retrieving data from session storage
let myData = sessionStorage.getItem('key');
// Deleting data from session storage
sessionStorage.removeItem('key');
// Clearing all data from session storage
sessionStorage.clear();
Discussing Common Errors and Debugging
When working with the Web Storage API, there are a couple of common errors you might run into. For example, you might hit the storage limit, or try to retrieve an item that doesn’t exist. It’s essential to anticipate these errors and handle them gracefully in your code.
A common way to handle errors is to check if an item exists before trying to retrieve it:
if (localStorage.getItem('key') !== null) {
// The item exists, do something with it
} else {
// The item does not exist
}
To handle hitting the storage limit, you could catch the error when trying to set an item:
try {
localStorage.setItem('key', 'value');
} catch (e) {
if (e.name === 'QuotaExceededError') {
// The storage limit has been exceeded, do something like clearing old data
}
}
You’ve made it through the hands-on part of the Web Storage API! As you can see, it’s a very straightforward API to use, but it offers a great deal of power and flexibility for enhancing your web applications. In the next section, we’ll take a look at how to ensure your usage of the Web Storage API is secure.
Security Considerations and Best Practices for Using Web Storage API
You’re now fully equipped with the knowledge of what the Web Storage API is, why it’s used, and how to use it. But like all powerful tools, it’s important to use the Web Storage API responsibly. In this section, we’re going to discuss how to ensure your use of the Web Storage API is secure and some best practices to follow.
Never Store Sensitive Information
One of the cardinal rules of using the Web Storage API is to never store sensitive information. This includes things like passwords, credit card numbers, and personally identifiable information. Why? Because data in web storage is accessible through JavaScript, which makes it susceptible to cross-site scripting (XSS) attacks. An attacker could potentially inject script into your site that reads web storage and sends the data back to them.
Always Validate and Sanitize Your Data
Just because data comes from your own site doesn’t mean it’s safe. If your website allows users to input data that gets stored in web storage, always validate and sanitize this data before using it. This can help protect against XSS attacks.
Using HTTPS
While the Web Storage API doesn’t inherently enforce the use of HTTPS, it’s strongly recommended to serve your site over HTTPS if you’re using web storage. This is especially true if your site is on the public internet. HTTPS encrypts the connection between the user’s browser and your server, making it harder for attackers to eavesdrop or tamper with the communication.
Regularly Clear Old Data
Remember that the Web Storage API has a limit on how much data it can store. As a best practice, regularly clear old or unneeded data from web storage. This not only helps manage storage limits, but can also improve performance and privacy.
Here’s an example of how you might clear old data from local storage:
// Let's say you're storing to-dos with a timestamp of when they were added
let todos = [
{ task: 'Buy milk', timestamp: 1626643200000 },
{ task: 'Walk the dog', timestamp: 1626729600000 },
// More to-dos...
];
// And you decide to-dos older than a week should be cleared
let oneWeekAgo = Date.now() - 7 * 24 * 60 * 60 * 1000;
todos = todos.filter(todo => todo.timestamp > oneWeekAgo);
localStorage.setItem('todos', JSON.stringify(todos));
Use a Library if Needed
If your needs are complex, consider using a library that abstracts some of the complexities of the Web Storage API. Libraries like localForage, store.js, and others offer additional features like storing other data types without needing to manually serialize them, handling storage limits, and more.
Using the Web Storage API responsibly is critical to maintaining a secure, efficient, and user-friendly website. By keeping these principles in mind, you’ll be well on your way to mastering this powerful tool!
And with that, we’ve covered all the key elements of the Web Storage API. Remember, like any tool, it’s all about choosing the right tool for the job. The Web Storage API is incredibly powerful for creating personalized, dynamic web experiences, but it’s just one of many tools available to you as a web developer. Happy coding!
Limitations of the Web Storage API
The Web Storage API is powerful, but like every tool, it has its limitations. It’s not always the best solution for every problem. Being aware of these limitations can help you make informed decisions about when and how to use the Web Storage API. Let’s discuss some of these limitations and possible ways around them.
Limited Storage Capacity
As we’ve mentioned earlier, the Web Storage API has a storage limit. While this limit is typically large enough for most uses (5-10MB depending on the browser), it can be a constraint for applications that need to store large amounts of data on the client side.
Only Stores Strings
The Web Storage API can only store data as strings. This means that you must serialize other types of data like arrays or objects before storing them, and then deserialize them when retrieving. This could potentially lead to performance issues if you’re working with large amounts of data.
Here’s an example of storing and retrieving an object:
// Here's an object we want to store
let user = {
name: 'Alice',
email: 'alice@example.com'
};
// We need to serialize it before storing
localStorage.setItem('user', JSON.stringify(user));
// And deserialize it when retrieving
let savedUser = JSON.parse(localStorage.getItem('user'));
No Expiry
Unlike cookies, data stored in web storage doesn’t have an expiry date. It stays in the user’s browser until it’s manually cleared, either by you through your code or by the user themselves. This could potentially lead to privacy concerns, and it also means you need to manage clearing old or unneeded data yourself.
Synchronous
The Web Storage API is synchronous, meaning it blocks the rest of your code from executing until it’s finished. This is usually not an issue because reading from and writing to web storage is very fast. However, if you’re storing large amounts of data, it could potentially lead to performance issues.
Not Accessible Across Different Domains
Data stored in web storage is isolated to the domain it was stored from. This means you can’t access data stored from example1.com
on example2.com
, even if they’re both your websites.
Alternatives to Web Storage API
If the limitations of the Web Storage API are a constraint for your specific use case, there are other options you might consider:
- IndexedDB: If you need to store large amounts of data on the client side, consider using IndexedDB. It’s a low-level API for client-side storage of significant amounts of structured data, including files and blobs.
- Cookies: While they have their own limitations, cookies could be a better choice if you need to store data that should be included with every server request, or if you need the data to expire after a certain period of time.
- Server-side storage: If client-side storage isn’t suitable, consider storing your data on the server side.
Understanding the limitations of the Web Storage API is crucial to using it effectively. By weighing these limitations against your specific needs and use cases, you can make the best choice for your application.
That brings us to the end of our deep dive into the Web Storage API. It’s been a lot of information, but with this knowledge, you’ll be able to harness the power of the Web Storage API to create dynamic, efficient, and user-friendly web applications.
The Future of Web Storage API
Before we wrap up, it’s worth considering the future of the Web Storage API. As the landscape of web development continually evolves, so do the technologies that support it. Let’s look at some potential developments and how they might affect the way we use web storage.
Increased Storage Limits
As web applications become more sophisticated, there’s a growing need for more client-side storage. While IndexedDB is currently the best choice for storing large amounts of data, we might see an increase in the storage limit of the Web Storage API in the future.
Enhanced Security
Security on the web is an ever-present and growing concern. As a result, browser vendors are constantly working to improve the security of web storage. We might see features in the future that mitigate some of the security concerns of using web storage, like better protection against XSS attacks.
Improved Performance
While the synchronous nature of the Web Storage API is rarely a problem, as web applications grow more complex, performance can become a concern. Future updates to the API could include asynchronous methods, similar to IndexedDB, to prevent potential blocking of the main thread.
Better Interoperability
Currently, data stored by the Web Storage API can’t be shared across different domains. While this is primarily a security feature, there could be future developments that allow for safe sharing of certain types of data across domains.
Greater User Control
Privacy is a significant concern for many web users. Future updates to the Web Storage API might give users more control over what data is stored and how long it’s kept. This could include clearer prompts about data storage or easier ways to manage and clear stored data.
Remember, the best way to stay ahead in the world of web development is to keep learning and adapting. As the Web Storage API and other web technologies evolve, so too should your skills and practices.
That’s it! You’ve come to the end of your deep dive into the Web Storage API. You now have the knowledge to use this powerful tool effectively, responsibly, and with an eye on the future. Here’s to creating amazing, dynamic web experiences.
Conclusion and Next Steps
Wow, what a journey it’s been! You’ve taken a deep dive into the world of the Web Storage API. From understanding what it is and why it’s used, to mastering how to use it and being aware of its limitations, you’re now ready to incorporate it into your web development toolkit.
Recap
Let’s take a quick moment to reflect on everything you’ve learned:
- What the Web Storage API is: You learned that the Web Storage API provides mechanisms for web applications to store data in a user’s browser.
- Why it’s used: You now understand that it’s used to create a seamless, personalized user experience by remembering users’ inputs, preferences, and previous activity.
- How to use it: You’ve practiced using the API’s methods to store, retrieve, and remove data, and to listen for storage events.
- Security considerations and best practices: You’ve noted the importance of data validation, sanitization, and serving your site over HTTPS. You know that you should never store sensitive information and that it’s a good idea to clear old data.
- Understanding its limitations: You’re aware that the API has a limited storage capacity, only stores strings, doesn’t have data expiry, is synchronous, and isolates data to specific domains.
- Looking to the future: You’ve speculated on possible future developments of the Web Storage API, including increased storage limits, enhanced security, improved performance, better interoperability, and greater user control.
Next Steps
It’s time for you to start experimenting with the Web Storage API on your own. Try implementing it in a project. Perhaps you could start with a simple to-do list where users can add and remove tasks, and their tasks will still be there when they come back later. Or maybe a customizable greeting message that remembers the user’s name.
Remember, the more you practice, the more comfortable you’ll become with the API. And if you ever get stuck or want to dive even deeper, there are plenty of resources available on the web to help you out.
The world of web development is constantly evolving, and learning to adapt and utilize these changes is a critical part of being a successful developer. Keep exploring, keep building, and most importantly, keep having fun while doing it.
Here’s to your journey in web development, and congratulations on adding another tool to your web development toolkit. Happy coding!
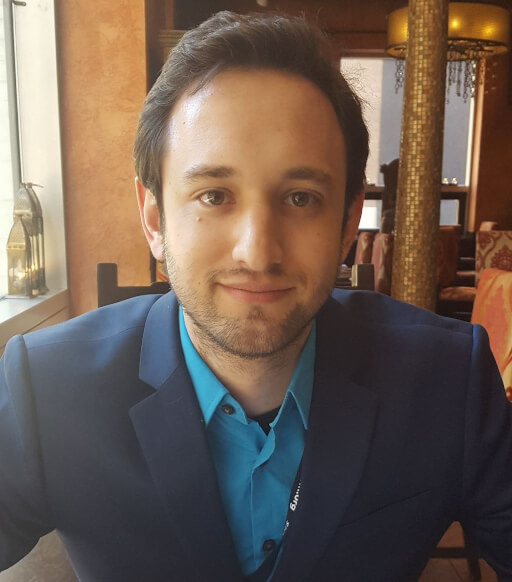
David Selden-Treiman is Director of Operations and a project manager at Potent Pages. He specializes in custom web crawler development, website optimization, server management, web application development, and custom programming. Working at Potent Pages since 2012 and programming since 2003, David has extensive expertise solving problems using programming for dozens of clients. He also has extensive experience managing and optimizing servers, managing dozens of servers for both Potent Pages and other clients.
Comments are closed here.