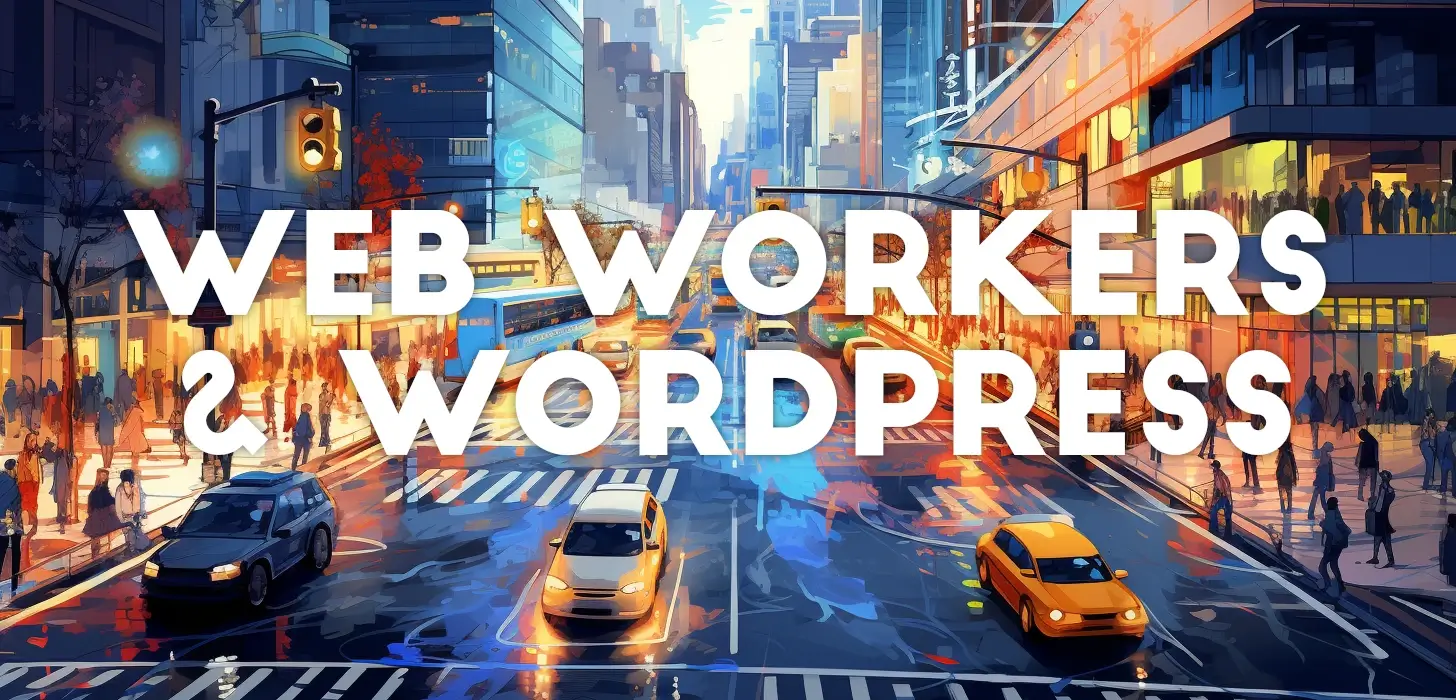
Web Workers: How To Improve WordPress Performance In 2025
April 12, 2023 | By David Selden-Treiman | Filed in: Website Speed.The TL-DR
Web Workers are a powerful technology that allows you to run computationally intensive tasks in separate threads, preventing your site’s main thread from being blocked and improving performance. To implement Web Workers in WordPress, you can create separate JavaScript files containing the worker code, instantiate them in your theme or plugin, and use postMessage() and onmessage event listeners to communicate between the main thread and Web Workers.
- Introduction
- Understanding Web Workers
- How Web Workers Can Enhance WordPress Performance
- Implementing Web Workers in WordPress
- Best Practices for Using Web Workers in WordPress
- Example Uses for Web Workers In WordPress
- Exploring Advanced Techniques and Tools
- Measuring the Impact of Web Workers on Your WordPress Site
- Common Challenges and Pitfalls When Implementing Web Workers
- Conclusion
Introduction
Hey there! If you’re managing a website, you probably already know that performance plays a crucial role in its success. A speedy website ensures that your visitors enjoy a smooth and seamless user experience, which in turn boosts user engagement and conversion rates. On top of that, search engines like Google factor in website speed when determining search rankings, so having a fast site can help you climb the SEO ladder.
Now, when it comes to building websites, WordPress is undoubtedly one of the most popular Content Management Systems (CMS) out there. You might be one of the millions of people who use WordPress to power your website, and it’s easy to see why. With its flexibility and extendibility, WordPress makes it simple for anyone to create and manage a site, even without extensive technical knowledge.
However, as your WordPress site grows and evolves, you might start to notice that it’s not as fast as it used to be. This is where performance optimization comes into play. By addressing common bottlenecks, such as slow-loading images or bulky scripts, you can ensure that your WordPress site remains speedy and responsive for all users.
One effective way to optimize your WordPress site’s performance is by leveraging Web Workers. In the following sections, we’ll dive deeper into what Web Workers are, how they can enhance your site’s performance, and how to implement them in your WordPress projects. So, buckle up, and let’s get started!
Understanding Web Workers
Before we jump into how Web Workers can improve your WordPress site, let’s take a moment to understand what they are and how they work. Simply put, Web Workers are a modern web technology that allows you to run JavaScript in the background, parallel to the main thread. This means that you can execute CPU-intensive tasks without causing your website to become unresponsive or slow down. Think of them as helpful little assistants that can take on some of the heavy lifting for you!
You can learn more about Web Workers from the Mozilla Developer Network.
Benefits of Using Web Workers
So, why should you consider using Web Workers for your WordPress site? Here are a couple of reasons:
Offloading Tasks From The Main Thread
By delegating time-consuming tasks to Web Workers, your main thread remains free to handle user interactions and render the UI. This leads to a more responsive and snappier website, especially when dealing with complex animations or data processing tasks.
Improved Performance And Responsiveness
With Web Workers running tasks in the background, your users will experience a smoother browsing experience, even on slower devices or network connections. This can be a game-changer, especially for mobile users who might not have access to high-performance hardware.
However, it’s important to note that Web Workers do come with some limitations. For instance, they can’t access the DOM directly, which means you’ll need to communicate with them through messages. This can be a bit tricky at first, but once you get the hang of it, it’s a powerful tool in your optimization arsenal.
Learn more about the limitations and considerations of Web Workers from this Google Web Fundamentals article.
Now that you have a better understanding of Web Workers and their benefits, let’s explore how they can be used to enhance your WordPress site’s performance.
How Web Workers Can Enhance WordPress Performance
Now that you’re familiar with Web Workers, let’s explore some specific ways they can boost your WordPress site’s performance. By offloading CPU-intensive tasks, handling large data sets, and parallelizing various operations, Web Workers can make a significant difference in your site’s speed and responsiveness.
Offloading CPU-Intensive Tasks
There are quite a few tasks in a typical WordPress site that can be resource-intensive, such as image manipulation, data processing, or complex calculations. By delegating these tasks to Web Workers, you can free up the main thread and ensure your site remains smooth and responsive.
For example, consider a WordPress site with a gallery plugin that loads high-resolution images. Resizing and optimizing these images on the main thread can slow down the page. By offloading these tasks to a Web Worker, you can improve the page load time and responsiveness.
Handling Large Data Sets
WordPress sites with large data sets, such as e-commerce stores or directory listings, can benefit greatly from Web Workers. Tasks like sorting, filtering, and searching through these data sets can be computationally expensive, especially on slower devices.
By delegating these tasks to Web Workers, you can provide a more seamless browsing experience for your users. For instance, if you have an e-commerce site with thousands of products, using Web Workers to perform search and filtering operations can significantly improve your site’s performance.
Parallelizing Tasks
Another advantage of Web Workers is their ability to run multiple tasks simultaneously. In a WordPress site, this can be particularly useful for optimizing the performance of plugins and themes that load multiple assets or execute numerous scripts.
For example, a complex WordPress theme with several stylesheets, scripts, and other assets can benefit from parallelizing the loading process using Web Workers. This can lead to faster page load times and a better user experience overall.
By understanding these use cases and integrating Web Workers into your WordPress site, you can significantly improve its performance and provide a better experience for your users. In the next sections, we’ll delve into the technical aspects of implementing Web Workers in WordPress.
Implementing Web Workers in WordPress
Now that you know the benefits of using Web Workers and how they can improve your WordPress site’s performance, it’s time to learn how to implement them. Don’t worry, it’s easier than you might think! Let’s go through the steps involved in creating a Web Worker and communicating with it.
Creating a Web Worker
To create a Web Worker, you’ll need to write a separate JavaScript file, known as the worker script. This script contains the code that will be executed by the Web Worker. It should include the logic for handling messages from the main thread and performing the necessary tasks.
For example, let’s say you want to create a Web Worker that handles image resizing. Your worker script might look something like this:
self.addEventListener('message', (event) => {
const { imageData, targetWidth, targetHeight } = event.data;
const resizedImage = resizeImage(imageData, targetWidth, targetHeight);
self.postMessage(resizedImage);
});
function resizeImage(imageData, targetWidth, targetHeight) {
// Code for resizing the image goes here
}
Once you’ve written the worker script, it’s time to register the Web Worker in your WordPress theme or plugin. In your main JavaScript file, you’ll create a new instance of the Worker object, passing the URL of your worker script as an argument:
const imageResizeWorker = new Worker('path/to/your/worker-script.js');
Communicating with Web Workers
With the Web Worker created and registered, the next step is to communicate with it. You’ll send messages to the worker using the postMessage method and receive messages from it using the onmessage event listener.
To send a message to the worker, call the postMessage method and pass the data you want to send:
const imageData = // Get image data from your page
const targetWidth = 300;
const targetHeight = 200;
imageResizeWorker.postMessage({ imageData, targetWidth, targetHeight });
To receive messages from the worker, add an onmessage event listener to the worker instance:
imageResizeWorker.onmessage = (event) => {
const resizedImage = event.data;
// Do something with the resized image, like updating the page
};
You should also handle any errors that might occur within the Web Worker by adding an onerror event listener:
imageResizeWorker.onerror = (error) => {
console.error('Error in Web Worker:', error);
};
That’s it! With these steps, you’ve successfully implemented a Web Worker in your WordPress site. Keep in mind that this is just a basic example – you can use Web Workers for various tasks, depending on your site’s needs and performance goals.
Best Practices for Using Web Workers in WordPress
Now that you know how to implement Web Workers in your WordPress site, it’s essential to follow some best practices to ensure you get the most out of them. By focusing on graceful degradation, monitoring and optimizing performance, and being mindful of security considerations, you can create a robust and efficient website.
Graceful Degradation
While most modern browsers support Web Workers, it’s essential to ensure that your site continues to function correctly for users with older or less capable browsers. To achieve this, you should implement a fallback mechanism that provides an alternative implementation for browsers that don’t support Web Workers.
For example, you could use feature detection to check if the user’s browser supports Web Workers, and if not, perform the task on the main thread:
if (typeof Worker !== 'undefined') {
// Create and use a Web Worker
} else {
// Perform the task on the main thread as a fallback
}
By ensuring graceful degradation, you’ll provide a consistent experience for all users, regardless of their browser or device capabilities.
Determine the Right Tasks to Offload
Not all tasks are suitable for Web Workers. It’s crucial to identify tasks that are computationally expensive or time-consuming, as these are the tasks that will benefit the most from being offloaded to a separate thread. Examples include data processing, file conversions, or complex calculations. Avoid offloading tasks that require direct access to the DOM or global objects, as Web Workers don’t have access to these resources.
Use a Modular and Decoupled Approach
Keep your Web Worker code modular and decoupled from the main thread to minimize the impact on overall code complexity. This makes it easier to maintain and update your site, as well as to reuse Web Worker code across different projects. Implementing Web Workers as separate scripts or using libraries that help with modularization, like workerize, can be beneficial.
Optimize Communication Between the Main Thread and Web Workers
Efficient communication between the main thread and Web Workers is essential for good performance. Use structured cloning or transferable objects to pass data between threads efficiently, and consider using libraries like Comlink to simplify the communication process. Additionally, try to minimize the number of messages passed between threads to reduce overhead.
Monitoring and Optimizing Web Worker Performance
To get the most out of Web Workers, you should keep an eye on their performance and optimize their execution. There are various profiling and debugging tools available, such as Chrome DevTools, that can help you identify bottlenecks and inefficiencies in your Web Workers.
By monitoring and optimizing your Web Workers, you can ensure they’re performing at their best and providing the performance improvements you’re aiming for.
Security Considerations
When using Web Workers, it’s essential to be mindful of security concerns, especially when handling sensitive data. As Web Workers run in a separate thread, they have their own scope and can’t access the DOM or other resources from the main thread. While this helps protect against certain attacks, it’s important to ensure that you’re not inadvertently exposing sensitive information through message-passing.
Additionally, be aware of cross-origin restrictions when using Web Workers, as they can only be created from the same origin as the main document. This helps prevent potential security issues related to cross-origin attacks.
By following these best practices, you can ensure that your implementation of Web Workers in WordPress not only improves performance but also remains secure and robust. With these guidelines in mind, you’ll be well on your way to optimizing your WordPress site and providing an excellent user experience for all your visitors.
Document Your Web Worker Implementations
Thoroughly document your Web Worker implementations to make it easier for other developers (or your future self) to understand and maintain your code. Clear documentation can be especially valuable when working with Web Workers, as their asynchronous nature and separate environment can make the code more challenging to understand at first glance.
Example Uses for Web Workers In WordPress
To help illustrate the impact Web Workers can have on WordPress performance, let’s take a look at a few real-world examples and case studies. These examples showcase how Web Workers have been used to solve performance challenges and enhance the user experience.
Example 1: Web Workers for image optimization in a gallery plugin
Imagine a WordPress site that relies heavily on visuals, such as a photography portfolio or an online art gallery. The site uses a gallery plugin to display high-resolution images in an organized and aesthetically pleasing manner. However, as more images are added, the site starts to slow down due to the time it takes to load and process these images.
By implementing a Web Worker, the gallery plugin can offload the image optimization tasks, such as resizing and compression, to the background. This allows the main thread to focus on rendering the UI and handling user interactions. As a result, the site’s performance improves, providing a smooth browsing experience even with a large number of images.
Example 2: Using Web Workers to speed up search in an e-commerce website
Consider an e-commerce website built on WordPress with thousands of products in its inventory. As users search and filter through the products, the site starts to lag due to the computational effort required to process the large data set.
In this scenario, Web Workers can be utilized to handle the search and filtering operations in the background. By offloading these tasks, the main thread remains free to render the UI and handle user interactions, leading to a more responsive and snappy browsing experience. This not only improves the site’s performance but also helps drive user engagement and conversions.
Example 3: Parallelizing asset loading in a complex WordPress theme
A WordPress site with a feature-rich theme may require loading multiple stylesheets, scripts, and other assets. Loading these assets sequentially can result in longer page load times, leading to a less-than-ideal user experience.
By using Web Workers, the theme’s asset loading process can be parallelized, allowing multiple assets to be loaded and processed simultaneously. This speeds up the page load time and ensures that the site remains responsive and visually appealing for users.
Example 4: Improving JavaScript-heavy plugins
Suppose you have a WordPress site with a plugin that performs complex animations or interactive visualizations, such as a data visualization plugin that displays graphs and charts. Running the calculations and animations on the main thread can cause the site to become unresponsive or slow.
By moving these resource-intensive tasks to a Web Worker, the plugin can continue to perform complex calculations and animations without affecting the site’s responsiveness. This ensures a smooth user experience, even when using plugins with heavy JavaScript workloads.
Example 5: Enhancing a live chat plugin’s performance
Imagine a WordPress site that includes a live chat plugin, allowing users to communicate with customer support or other users in real-time. As the number of active chat participants increases, the plugin’s performance may degrade due to the need to constantly update the chat messages and manage the user interface.
By implementing Web Workers, the chat plugin can offload tasks like message processing, filtering, and sorting to a separate thread. This allows the main thread to focus on rendering the chat UI and handling user input, leading to a more responsive and enjoyable chat experience for users.
Example 6: Optimizing a social media feed plugin
Let’s say your WordPress site uses a plugin that pulls in and displays social media feeds from various platforms. Parsing, formatting, and rendering these feeds on the main thread can slow down your site, especially if the plugin retrieves and processes large amounts of data.
In this case, you can use Web Workers to handle the data retrieval, parsing, and formatting tasks in the background. This frees up the main thread to focus on rendering the social media feeds and handling user interactions, resulting in a more responsive and engaging site.
Example 7: Speeding up a language translation plugin
Consider a WordPress site with a language translation plugin that allows users to view the site’s content in their preferred language. Translating the content in real-time on the main thread can be resource-intensive, particularly if the site contains a large amount of text or uses complex translation algorithms.
By offloading the translation tasks to a Web Worker, the plugin can perform translations in the background without affecting the site’s responsiveness. This allows users to seamlessly switch between languages and enjoy a smooth browsing experience, regardless of the complexity of the translation process.
Example 8: Offloading geolocation calculations for a mapping plugin
Imagine a WordPress site that utilizes a mapping plugin to display various locations, such as a real estate site showcasing properties or a travel blog with interactive maps. Performing geolocation calculations and map updates on the main thread can consume considerable resources, leading to a sluggish user experience.
By employing Web Workers, you can offload geolocation calculations, distance measurements, and map rendering tasks to the background. This allows the main thread to focus on user interactions and maintain a responsive, snappy browsing experience for your visitors.
Example 9: Enhancing performance for a weather forecasting plugin
Consider a WordPress site with a weather forecasting plugin that retrieves and displays real-time weather data for multiple locations. Processing and updating this data on the main thread can slow down your site, especially if the plugin retrieves large datasets or updates frequently.
By using Web Workers, you can delegate the tasks of fetching, processing, and updating weather data to the background. This frees up the main thread to handle user interactions and render the weather information, resulting in a more responsive and engaging experience for your users.
Example 10: Optimizing a text analysis plugin for blog authors
Suppose you have a WordPress site with a text analysis plugin that helps blog authors improve their writing by offering suggestions, such as grammar corrections, readability scores, or keyword analysis. Analyzing text on the main thread can be resource-intensive, particularly for lengthy articles or in-depth analysis.
In this case, Web Workers can be used to offload the text analysis tasks to a separate thread, allowing the main thread to focus on updating the author’s writing interface and handling user input. This ensures a smooth writing experience for authors, even when using advanced text analysis features.
Example 11: Speeding up a file conversion plugin
Imagine a WordPress site with a plugin that allows users to upload and convert various file formats, such as images, documents, or audio files. Processing and converting these files on the main thread can be time-consuming and may cause the site to become unresponsive.
By moving the file conversion tasks to a Web Worker, you can perform these operations in the background without affecting the site’s responsiveness. This enables users to continue browsing the site while their files are being converted, providing a seamless and efficient user experience.
These examples highlight the potential benefits of using Web Workers in various WordPress scenarios. By understanding these use cases and applying Web Workers to your projects, you can significantly improve your site’s performance and deliver a better experience to your users.
Exploring Advanced Techniques and Tools
As you become more comfortable with using Web Workers in your WordPress projects, you might be interested in exploring advanced techniques and tools to further enhance your site’s performance. Let’s take a look at some options that can help you take your Web Worker implementations to the next level.
Shared Web Workers
While regular Web Workers are tied to a single page, Shared Web Workers can be shared across multiple pages or even across multiple instances of the same page. This can be beneficial for tasks that need to be synchronized across different parts of your site or for conserving resources when multiple pages are open simultaneously.
For example, imagine a WordPress site with a live chat plugin that users can access from multiple pages. By using a Shared Web Worker, the chat plugin can maintain a single chat instance and synchronize messages across all open pages, providing a seamless and consistent chat experience for users.
Web Worker Libraries and Frameworks
Several libraries and frameworks can help you manage Web Workers more efficiently and simplify their implementation. Some popular options include:
Comlink: A library that simplifies the communication between the main thread and Web Workers by using a more intuitive interface for message passing.
Greenlet: A library that allows you to run inline functions as Web Workers, making it easy to offload specific tasks without having to create separate worker scripts.
workerize: A library that enables you to move a module into a Web Worker, making it simple to offload entire modules without having to rewrite your code.
By leveraging these libraries and frameworks, you can streamline your Web Worker implementations and focus on improving your site’s performance.
Service Workers
Service Workers are a type of Web Worker that act as a proxy between your web app and the network. They enable advanced features such as offline support, background sync, and push notifications. While they serve a different purpose than traditional Web Workers, they can be used alongside them to further enhance your site’s performance and user experience.
For example, a WordPress site with a news plugin could use a Service Worker to cache and serve articles offline, ensuring that users can still access content even without an active internet connection. This not only improves the site’s performance but also makes it more resilient and accessible for users with unreliable network connections.
By exploring these advanced techniques and tools, you can further optimize your WordPress site’s performance and create an even more engaging and enjoyable experience for your users. Embracing these advanced options will help you stay ahead of the curve and ensure that your site remains competitive in the ever-evolving digital landscape.
Measuring the Impact of Web Workers on Your WordPress Site
As you implement Web Workers to enhance your WordPress site’s performance, it’s crucial to measure their impact to ensure that your optimizations are delivering the desired results. In this section, we’ll discuss some key performance indicators (KPIs) and tools that can help you evaluate the effectiveness of your Web Worker implementations.
Key Performance Indicators (KPIs)
When assessing the impact of Web Workers, you’ll want to monitor several KPIs, including:
- Page load time: Measure how long it takes for your site to load, both with and without Web Workers. A reduction in page load time indicates a positive impact on site performance.
- Time to Interactive (TTI): This metric tracks the time it takes for a page to become fully interactive. A lower TTI suggests that Web Workers are effectively offloading tasks and allowing the main thread to focus on user interactions.
- First Contentful Paint (FCP): FCP measures the time it takes for the first piece of content to appear on the screen. An improvement in FCP indicates that Web Workers are helping to render content more quickly.
By tracking these KPIs, you can gain a better understanding of how Web Workers are impacting your site’s performance and make adjustments as needed to optimize their implementation.
Performance Measurement Tools
There are several tools available to help you measure the performance of your WordPress site and assess the impact of Web Workers. A couple popular options include:
- Google Lighthouse: Lighthouse is an open-source tool that provides a comprehensive performance audit for your site, including metrics such as page load time, TTI, and FCP. You can use Lighthouse to compare your site’s performance with and without Web Workers, giving you valuable insights into their impact.
- Chrome DevTools: As mentioned earlier, Chrome DevTools is a powerful set of tools for debugging and profiling your site. You can use the Performance tab in DevTools to measure key performance metrics and analyze the impact of Web Workers on your site’s responsiveness.
By using these tools and monitoring the relevant KPIs, you can effectively measure the impact of Web Workers on your WordPress site’s performance. This information will help you fine-tune your implementations, ensuring that you maximize the benefits of Web Workers and provide the best possible user experience for your visitors.
Common Challenges and Pitfalls When Implementing Web Workers
As you begin to implement Web Workers in your WordPress projects, you might encounter some common challenges and pitfalls. Being aware of these issues can help you navigate them more effectively and ensure a smoother integration process. Let’s discuss some of the most common challenges and how to overcome them.
Challenge 1: Limited Access to the DOM and Global Objects
Web Workers run in a separate environment from the main thread and don’t have direct access to the DOM or global objects like window and document. This can be a challenge when attempting to offload tasks that rely on these objects.
Solution: To work around this limitation, you can pass the required data to the Web Worker as messages and return the results to the main thread for DOM manipulation. For complex interactions, consider using libraries like Comlink to simplify the communication process between the main thread and Web Workers.
Challenge 2: Managing Communication Between Main Thread and Web Workers
Passing messages between the main thread and Web Workers can be cumbersome, especially when dealing with complex data structures or large amounts of data.
Solution: Use structured cloning or transferable objects to efficiently pass data between the main thread and Web Workers. Structured cloning allows you to copy complex data structures, while transferable objects enable you to pass large data buffers without copying. Additionally, libraries like Comlink can help simplify the communication process.
Challenge 3: Debugging Web Workers
Debugging Web Workers can be more challenging than debugging code running on the main thread, as they run in a separate environment and have different debugging tools.
Solution: Use browser developer tools, like Chrome DevTools, to debug Web Workers. Most browsers provide dedicated tools to inspect and debug Web Workers, allowing you to set breakpoints, step through code, and inspect variables. Familiarize yourself with the debugging tools available for your preferred browser to make the debugging process smoother.
Challenge 4: Browser Compatibility
While Web Workers are widely supported across modern browsers, some older browsers may not support them, potentially causing issues for users on outdated software.
Solution: Check for Web Worker support using feature detection and provide fallback solutions for browsers that don’t support them. You can use a tool like caniuse.com to verify browser compatibility and plan your implementations accordingly.
Challenge 5: Increased Complexity
Integrating Web Workers into your WordPress projects can increase code complexity, making it more challenging to maintain and update your site.
Solution: Keep your Web Worker implementations as modular and decoupled as possible to minimize the impact on overall code complexity. Additionally, consider using libraries and frameworks that simplify Web Worker management, such as Comlink, Greenlet, or workerize.
By understanding these common challenges and preparing for them, you can ensure a more successful integration of Web Workers into your WordPress projects. This will help you reap the benefits of improved performance while minimizing potential issues along the way.
Conclusion
In conclusion, leveraging Web Workers can significantly enhance your WordPress site’s performance by offloading computationally expensive tasks to separate threads. By identifying the right tasks to offload, following best practices, and utilizing the available tools and libraries, you can create a smoother and more responsive user experience for your site visitors.
Remember to measure the impact of your Web Worker implementations using performance measurement tools like Google Lighthouse and Chrome DevTools. This will help you fine-tune your optimizations and ensure that you’re making the most of this powerful technology.
As you embark on your Web Worker journey, keep in mind the common challenges and pitfalls, and be prepared to overcome them. By doing so, you’ll be well-equipped to harness the full potential of Web Workers and take your WordPress site’s performance to new heights.
Now it’s time to get started! Dive into the world of Web Workers, explore the various techniques and tools available, and create a faster, more efficient WordPress site that delights your users and keeps them coming back for more.
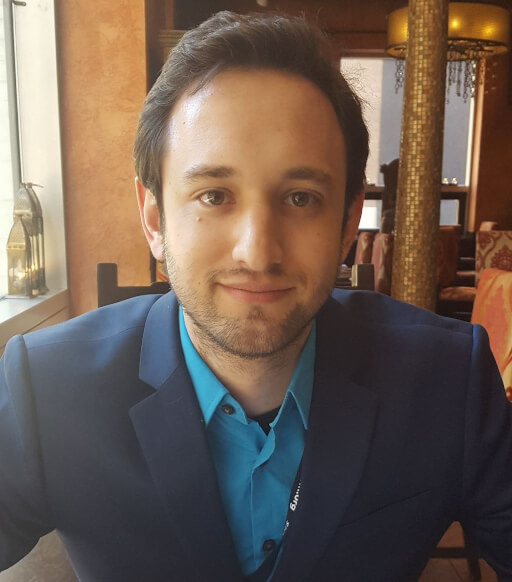
David Selden-Treiman is Director of Operations and a project manager at Potent Pages. He specializes in custom web crawler development, website optimization, server management, web application development, and custom programming. Working at Potent Pages since 2012 and programming since 2003, David has extensive expertise solving problems using programming for dozens of clients. He also has extensive experience managing and optimizing servers, managing dozens of servers for both Potent Pages and other clients.
Comments are closed here.